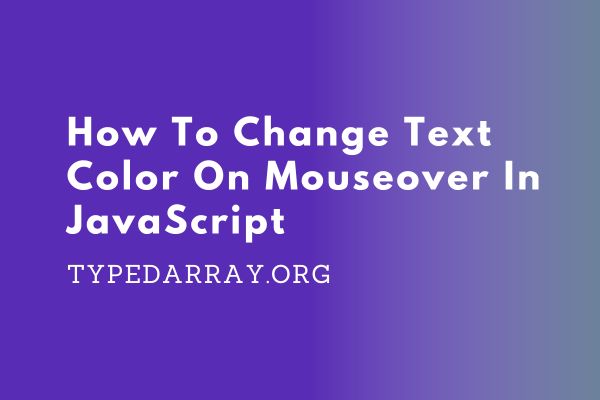
In order to change text color on mouseover in JavaScript, you will need to create an event listener on the mouseover event. This can be accomplished by creating a function that listens for the event and then changes the CSS property of the text.
How To Change Text Color On Mouseover In JavaScript
Learn how to add a dynamic touch to your website by changing text color on mouseover using JavaScript. This comprehensive guide provides step-by-step instructions and code snippets to help you achieve this interactive effect effortlessly.
Step 1: Create A Function For Color Change
The code for this would look something like this:
function changeColor(elem,color) {
elem.style.color = color;
}
The style.color property is what controls the text color of an element. In order to change this property, you will need to use a function that takes in an element and a color as parameters. This function will then change the text color of the element to the specified color.
Step 2: Apply The Event Listener
You can then add this event listener to any element on your page, like so:
document.getElementById("myDiv").addEventListener("mouseover", function handleMouseOver() {
changeColor(this, "red");
});
This code will automatically cause the element with an ID of “myDiv” to change its text color to red whenever it is hovered over. You can use this technique to create a variety of different effects, such as highlighting text or changing the color when clicked.
Step 3: Revert To Original Color On Mouseout
You can use the mouseout event to change the color back to its original state:
document.getElementById("myDiv").addEventListener("mouseout", function handleMouseOut() {
changeColor(this, "black");
});
This code will cause the element with an ID of “myDiv” to change its text color back to black when the mouse is no longer hovering over it.
Taking It Further: Changing Background Color
You can also use the same technique to change the background color of an element on mouseover. Just replace the “color” property in the CSS with “background-color”. For example:
function changeColor(elem,color) {
elem.style.backgroundColor = color;
}
The style.backgroundColor property is what controls the background color of an element. In order to change this property, you will need to use a function that takes in an element and a color as parameters. This function will then change the background color of the element to the specified color.
Applying Event Listeners For Background Color
Implement the event listeners for background color changes, mirroring the steps for text color changes:
document.getElementById("myDiv").addEventListener("mouseover", function handleMouseOver() {
changeColor(this, "red");
});
document.getElementById("myDiv").addEventListener("mouseout", function handleMouseOut() {
changeColor(this, "black");
});
Customizing For Different Elements
You can change the color of any other element as well, not necessarily the one on which the getElementById() function is called. For example, if you wanted to change the text color of an element div with the ID “myOtherDiv”, you could simply use this code:
document.getElementById("myDiv").addEventListener("mouseover", function handleMouseOver() {
changeColor(myOtherDiv, "red");
});
This function will change the text color of “myOtherDiv” to red when the user mouses over “myDiv”.
And then the event listener for mouseout would look like this:
document.getElementById("myDiv").addEventListener("mouseout", function handleMouseOut() {
changeColor(myOtherDiv, "black");
});
This function will change the text color of “myOtherDiv” back to black when the user mouses out of “myDiv”.
Conclusion – Change Text Color On Mouseover In JavaScript
Transform your website with dynamic effects that are sure to impress your visitors. Take the first step towards interactivity by implementing text and background color changes on mouseover using JavaScript. Try it out on your own site today!