To count the duplicates in an array in JavaScript, you can use any of the following methods –
- Use the forEach() method to iterate over the array, and on each iteration increment the count of the elements.
- Use the reduce() method to iterate over the array, and on each iteration increment the count of the elements.
Let’s discuss these methods in detail below.
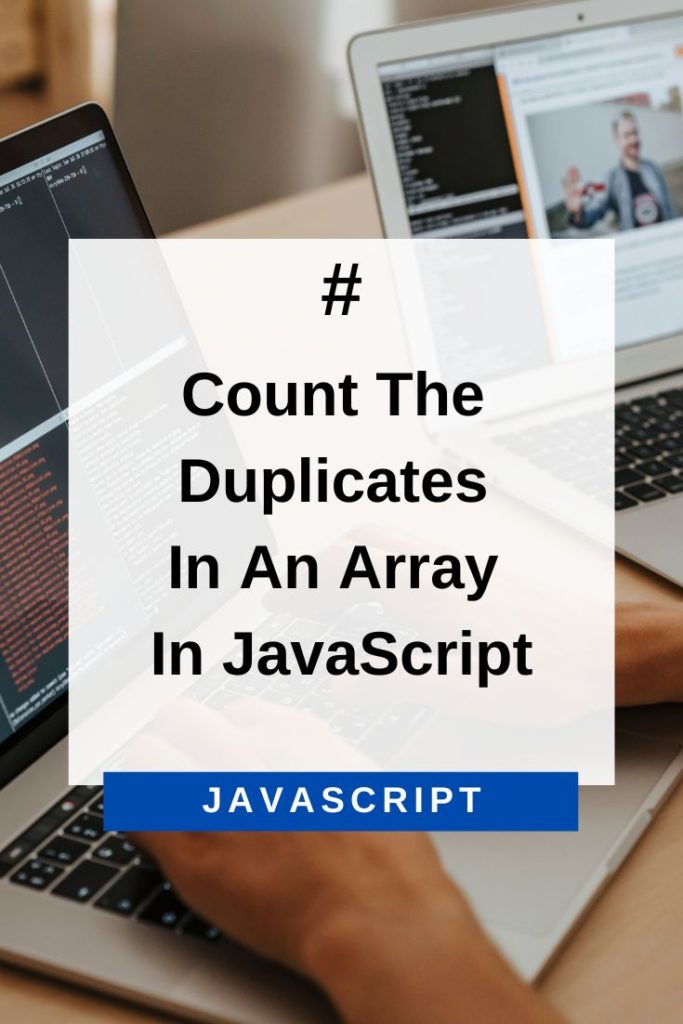
forEach() Method To Count The Duplicates In An Array In JavaScript
The forEach() method is used to iterate over an array in JavaScript. It takes a callback function as an argument, which is executed on each iteration.
We can use the forEach() method to count the duplicates in an array in JavaScript by creating an object called count, and incrementing the count of each element on each iteration.
let count = {};
function countDuplicates(arr) {
arr.forEach(function(element) {
if (!count.hasOwnProperty(element)) { //or (!count[element])
count[element] = 1;
} else {
count[element]++;
}
});
}
countDuplicates([1,2,3,1,4,5,3]);
console.log(count);
This will give us the output – { ‘1’: 2, ‘2’: 1, ‘3’: 2, ‘4’: 1, ‘5’: 1 }.
We can get the duplicates, i.e., elements that have a count >1, from the output of the code above by using the filter() method.
let duplicates = Object.keys(count).filter((element) => count[element] > 1);
console.log(duplicates);
This will give us the output – [‘1′,’3’].
In the above code, we have first created an object called count, which will store the count of each element in the array.
Then we have used the forEach() method to iterate over the array. On each iteration, we check if the element is already present in the object or not. If it is not present, then we add it to the object with the value 1. If it is already present, then we increment its value by 1.
At the end, we print the count object to see the number of times each element occurs in the array. If an element occurs more than 1 times, we count it as a duplicate and add it to the array called duplicates.
reduce() Method To Count The Duplicates In An Array In JavaScript
The reduce() method is used to iterate over an array in JavaScript. It takes a callback function as an argument, which is executed on each iteration.
We can use the reduce() method to count the duplicates in an array in JavaScript by creating an object called count, and incrementing the count of each element on each iteration.
let count = {};
function countDuplicates(arr) {
return arr.reduce(function(acc, element) {
if (!acc.hasOwnProperty(element)) { //or (!acc.[element])
acc[element] = 1;
} else {
acc[element]++;
}
return acc;
}, {});
}
count = countDuplicates([1,2,3,1,4,5,3]);
console.log(count);
This will give us the output – { ‘1’: 2, ‘2’: 1, ‘3’: 2, ‘4’: 1, ‘5’: 1 }.
We can get the duplicates, i.e., elements that have a count >1, from the output of the code above by using the filter() method.
let duplicates = Object.keys(count).filter((element) => count[element] > 1);
console.log(duplicates);
This will give us the output – [‘1′,’3’].
In the above code, we have first created an object called count, which will store the count of each element in the array.
Then we have used the reduce() method to iterate over the array. On each iteration, we check if the element is already present in the acc object or not. If it is not present, then we add it to the object with the value 1. If it is already present, then we increment its value by 1.
At the end, we print the count object to see the number of times each element occurs in the array. If an element occurs more than 1 times, we count it as a duplicate and add it to the array called duplicates.
We can also use the destructuring assignment syntax to count the duplicates in an array in JavaScript.
let count = {};
function countDuplicates(arr) {
count = arr.reduce((accumulator, value) => {
return {…accumulator, [value]: (accumulator[value] || 0 )+ 1};
},{});
}
countDuplicates([1,2,3,1,4,5,3]);
console.log(count);
This will give us the output – { ‘1’: 2, ‘2’: 1, ‘3’: 2, ‘4’: 1, ‘5’: 1 }.
We can get the duplicates, i.e., elements that have a count >1, from the output of the code above by using the filter() method.
let duplicates = Object.keys(count).filter((element) => count[element] > 1);
console.log(duplicates);
This will give us the output – [‘1′,’3’].
In the above code, we have used the destructuring assignment syntax to create an object called count, which will store the count of each element in the array.
Then we have used the reduce() method to iterate over the array. On each iteration, we check if the element is already present in the object or not. If it is not present, then we add it to the object with the value 1. If it is already present, then we increment its value by 1.
At the end, we print the count object to see the number of times each element occurs in the array. If an element occurs more than 1 times, we count it as a duplicate and add it to the array called duplicates.
Conclusion
In this article, we have seen how to count the duplicates in an array in JavaScript using various methods.
We can use either the reduce() method or the forEach() method to create an object that stores the count of each element in the array.
Then we can use the filter() method to get the elements that have a count more than 1.
I hope this article has been helpful to you. Thank you for reading!