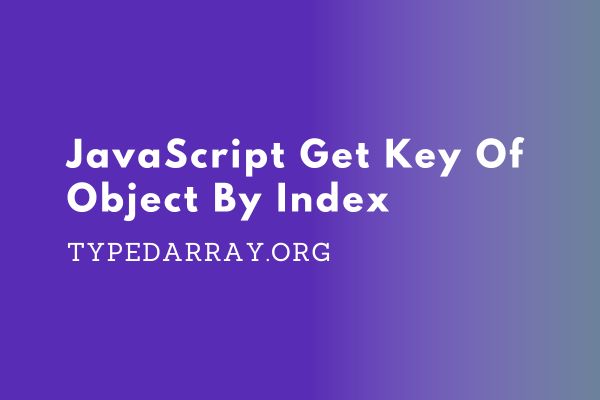
In JavaScript, objects are widely used to store and manipulate data. While accessing the values of an object is straightforward, obtaining the corresponding key by its index can be a bit trickier.
However, there are several methods available that allow you to achieve this task efficiently. By utilizing these techniques, you can access the keys of an object using their respective indices, providing flexibility and versatility in your JavaScript code.
In this article, we will explore various approaches to obtaining the key of an object by index, enabling you to retrieve specific keys based on their position within the object. Let’s dive into the different methods available for accomplishing this in JavaScript.
JavaScript Get Key Of Object By Index
There are various ways to get the key of an object in Javacript –
- Use
Object.keys()
to get an array of keys and access the key by index. - Use a
for...in
loop to iterate over the object and track the index to get the key. - Use
Object.entries()
to get an array of key-value pairs and access the key by index. - Convert the object to an array using
Object.getOwnPropertyNames()
and access the key by index. - Use
Reflect.ownKeys()
to get an array of keys and access the key by index. - Use the
Map
object instead of a regular object, which allows direct access to keys by index usingMap.prototype.get()
.
Please note that while these methods can be used to get the key of an object by index in JavaScript, they may have different behaviors and considerations based on the specific use case.
Use Object.keys()
One way to obtain the key of an object by index in JavaScript is by using the Object.keys()
method. The Object.keys()
method returns an array containing the enumerable property names of an object. By accessing the array element at a specific index, you can retrieve the key associated with that index.
Here’s an example that demonstrates the usage of Object.keys()
to get the key of an object by index:
const obj = { name: "John", age: 30, city: "New York" };
const keys = Object.keys(obj);
// Accessing the key at index 1
const keyAtIndex1 = keys[1];
console.log(keyAtIndex1); // Output: age
In the above code snippet, we have an object obj
with three properties: name
, age
, and city
. By calling Object.keys(obj)
, we obtain an array containing the keys of the object. We then access the key at index 1 using keys[1]
, which gives us the key "age"
. By utilizing Object.keys()
, you can retrieve the key of an object by its corresponding index.
Use a for…in loop
Another way to retrieve the key of an object by index in JavaScript is by utilizing a for...in
loop. The for...in
loop allows you to iterate over the enumerable properties of an object, providing access to each key within the loop’s body. By keeping track of the index while iterating, you can retrieve the key associated with a specific index.
Here’s an example that demonstrates the use of a for...in
loop to get the key of an object by index:
const obj = { name: "John", age: 30, city: "New York" };
let index = 0;
let keyAtIndex = "";
for (let key in obj) {
if (index === 1) {
keyAtIndex = key;
break;
}
index++;
}
console.log(keyAtIndex); // Output: age
In the above code snippet, we have an object obj
with three properties: name
, age
, and city
. The for...in
loop iterates over each property of the object. We use an index
variable to keep track of the current index while iterating.
When the desired index (in this case, 1) is reached, we assign the corresponding key to the keyAtIndex
variable and exit the loop using break
.
In this example, the key at index 1 is "age"
. By using a for...in
loop, you can retrieve the key of an object by its associated index.
Use Object.entries()
One more approach to obtain the key of an object by index in JavaScript is by utilizing the Object.entries()
method. The Object.entries()
method returns an array of key-value pairs, where each pair is represented as an array itself. By accessing the element at a specific index in the resulting array, you can retrieve the key associated with that index.
Here’s an example that demonstrates the usage of Object.entries()
to get the key of an object by index:
const obj = { name: "John", age: 30, city: "New York" };
const entries = Object.entries(obj);
// Accessing the key at index 1
const keyAtIndex1 = entries[1][0];
console.log(keyAtIndex1); // Output: age
In the above code snippet, we have an object obj
with three properties: name
, age
, and city
. By calling Object.entries(obj)
, we obtain an array of key-value pairs: [[key1, value1], [key2, value2], ...]
. We then access the key at index 1 by using entries[1][0]
, which gives us the key "age"
. By utilizing Object.entries()
, you can retrieve the key of an object by its corresponding index in a concise manner.
Using Object.getOwnPropertyNames()
To retrieve the key of an object by index in JavaScript, you can use the Object.getOwnPropertyNames()
method to convert the object’s properties into an array. Once the object is converted to an array, you can access the key by its corresponding index.
Here’s an example that demonstrates the usage of Object.getOwnPropertyNames()
to get the key of an object by index:
const obj = { name: "John", age: 30, city: "New York" };
const keys = Object.getOwnPropertyNames(obj);
// Accessing the key at index 1
const keyAtIndex1 = keys[1];
console.log(keyAtIndex1); // Output: age
In the above code snippet, we have an object obj
with three properties: name
, age
, and city
. By calling Object.getOwnPropertyNames(obj)
, we obtain an array containing the property names of the object.
We then access the key at index 1 using keys[1]
, which gives us the key "age"
.
By utilizing Object.getOwnPropertyNames()
, you can convert the object’s properties into an array and retrieve the key of the object by its corresponding index.
Use Reflect.ownKeys()
To retrieve the key of an object by index in JavaScript, you can use the Reflect.ownKeys()
method. This method returns an array of all own property keys (both enumerable and non-enumerable) of an object. By accessing the element at a specific index in the resulting array, you can retrieve the key associated with that index.
Here’s an example that demonstrates the usage of Reflect.ownKeys()
to get the key of an object by index:
const obj = { name: "John", age: 30, city: "New York" };
const keys = Reflect.ownKeys(obj);
// Accessing the key at index 1
const keyAtIndex1 = keys[1];
console.log(keyAtIndex1); // Output: age
In the above code snippet, we have an object obj
with three properties: name
, age
, and city
. By calling Reflect.ownKeys(obj)
, we obtain an array containing all the own property keys of the object.
We then access the key at index 1 using keys[1]
, which gives us the key "age"
. By utilizing Reflect.ownKeys()
, you can retrieve the key of an object by its corresponding index, including both enumerable and non-enumerable properties.
Use the Map
object instead of a regular object
If you want to retrieve the key of an object by index in JavaScript, you can consider using the Map
object instead of a regular object. The Map
object provides a data structure that allows direct access to keys by index using the Map.prototype.get()
method.
Here’s an example that demonstrates the usage of the Map
object to get the key by index:
const map = new Map();
map.set("name", "John");
map.set("age", 30);
map.set("city", "New York");
// Accessing the key at index 1
const keyAtIndex1 = Array.from(map.keys())[1];
console.log(keyAtIndex1); // Output: age
In the above code snippet, we create a Map
object and populate it with key-value pairs. We then convert the keys of the Map
object into an array using Array.from(map.keys())
. By accessing the element at index 1 in the resulting array, we retrieve the key "age"
.
By using the Map
object, you can directly access keys by index using Array.from(map.keys())[index]
or by iterating over the Map
object using a loop and tracking the index. This provides a convenient way to retrieve the key of an object by index in JavaScript.
Conclusion
Retrieving the key of an object by index in JavaScript is a common requirement in various programming scenarios. Throughout this discussion, we explored several methods to accomplish this task efficiently.
We started by using Object.keys()
, which returns an array of keys and allows us to access the key by index. We then examined the for...in
loop, which enables us to iterate over the object’s properties and retrieve the key by tracking the index within the loop.
Additionally, we explored the Object.entries()
method, which converts the object into an array of key-value pairs. By accessing the desired index in the resulting array, we could extract the corresponding key.
Another approach involved utilizing Object.getOwnPropertyNames()
, which returns an array of the object‘s property names. We could then access the key by index in this array.
Lastly, we discussed the option of using the Reflect.ownKeys()
method, which returns an array of all own property keys of an object, including both enumerable and non-enumerable properties. By accessing the key at a specific index in this array, we could retrieve the desired key.
Additionally, we mentioned the alternative of using the Map
object, which allows direct access to keys by index using the Map.prototype.get()
method. By converting the Map
object’s keys into an array, we could access the key at a specific index.
Overall, the choice of method depends on the specific requirements and constraints of your JavaScript project. By understanding these various approaches, you can confidently retrieve the key of an object by index, facilitating effective data manipulation and processing in your JavaScript applications.