To check how many times an element appears in a JavaScript array –
- Declare a count variable and initialize it to 0.
- Loop through the array, For each element in the array, check if the current element is equal to the given element. If it is, increment the count variable by 1. After the loop, return the count variable.
Let’s discuss this method in detail below.
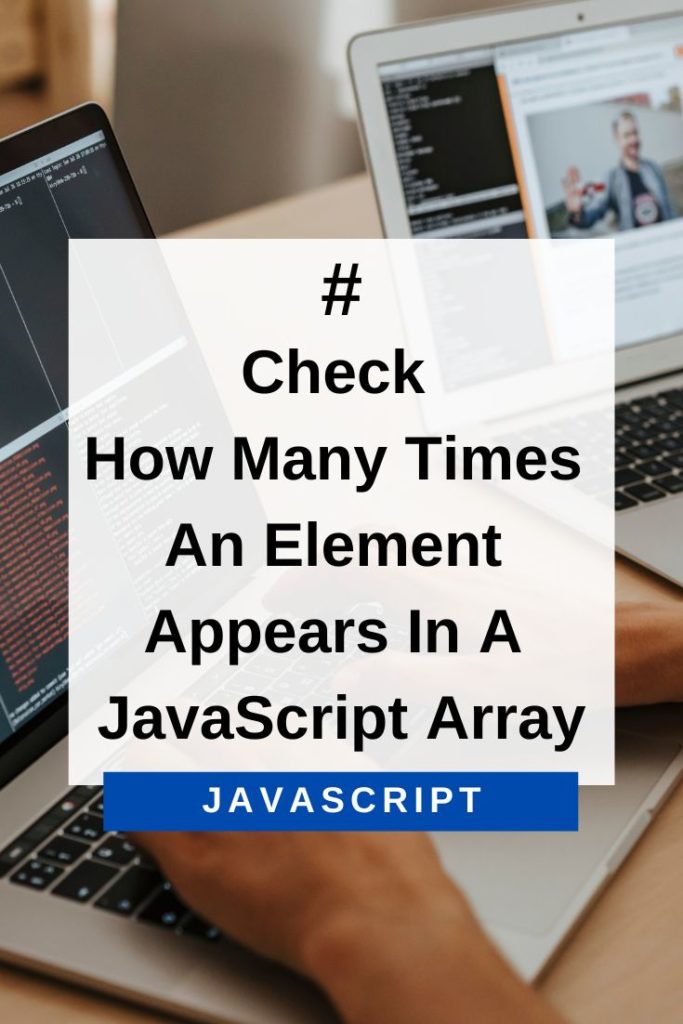
Check How Many Times An Element Appears In A JavaScript Array
Consider the following array:
var myArray = ['a', 'b', 'b', 'a', 'b'];
In the above array, the element “a” appears two times while the element “b” appears thrice.
To check how many times an element X appears in the array, we need to loop through the array and compare each element with X. If the current element is equal to X, we need to increment a counter variable by 1. After the loop ends, we can return this counter variable.
Using forEach() Method
Here is the code using forEach() method:
var myArray = ['a', 'b', 'b', 'a', 'b'];
var count = 0;
myArray.forEach(function(value) {
if (value === 'a') {
count++;
}
});
console.log(count); // 2
As you can see, we have declared a count variable and initialized it to 0. We are using forEach() method to loop through each element in the array. For each element, if the value is equal to “a”, we are incrementing the count variable by 1. Finally, we are logging the value of count which is 2.
Using for Loop
You can also use the for loop as follows:
var myArray = ['a', 'b', 'b', 'a', 'b'];
var count = 0;
for (var i = 0; i < myArray.length; i++) {
if (myArray[i] === 'a') {
count++;
}
}
console.log(count); // 2
In the above code, we are using a for loop to loop through each element in the array. For each element, if it is equal to “a”, we are incrementing the count variable by 1. Finally, we are logging the value of count which is 2.
Using filter() Method
You can also use the filter() method as follows:
var myArray = ['a', 'b', 'b', 'a', 'b'];
var count = myArray.filter(function(value) {
return value === 'a';
}).length;
console.log(count); // 2
In the above code, we are using the filter() method to create a new array which contains only those elements from the original array which are equal to “a”. The length property of this new array gives us the count of how many times “a” appears in the original array.
Using reduce() Method
You can also use the reduce() method as follows:
var myArray = ['a', 'b', 'b', 'a', 'b'];
var count = myArray.reduce(function(acc, value) {
if (value === 'a') {
return acc + 1;
} else {
return acc;
}
}, 0);
console.log(count); // 2
In the above code, we are using the reduce() method to loop through each element in the array. For each element, if it is equal to “a”, we are incrementing the accumulator variable by 1.
The reduce() method takes an accumulator variable and an array as arguments. The accumulator variable is used to store the intermediate results and it is initialized with the value passed as the second argument to the reduce() method. In our case, we have initialized it with 0. Finally, we are logging the value of count which is 2.
Conclusion
In this article, you have learned how to check how many times an element appears in a JavaScript array. There are several ways to do it and you have learned all of them. You can choose the method based on your requirements.