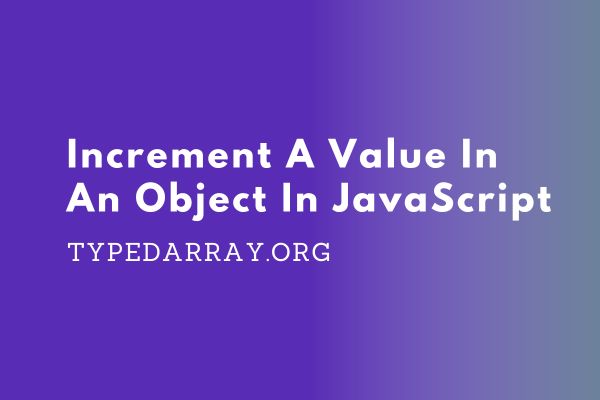
There are several ways to increment a value in an object in JavaScript. You can use dot notation, bracket notation, explicit value assignment, the +=
operator, the ++
operator, as well as nullish coalescing (??
) and logical OR (||
) operators.
These methods provide flexibility depending on your specific needs. Whether you want to increment a numerical property or provide a default value before incrementing, these approaches offer different ways to achieve your desired outcome. Choose the method that best suits your object structure and requirements.
Increment A Value In An Object In JavaScript
There are many ways to increment a value in an object in JavaScript. Some of these ways are –
- Dot notation
- Bracket notation
- Nullish coalescing (
??
) - Logical OR (
||
) operator
Let’s discuss each of these approaches in detail below.
Dot Notation To Increment A Value In An Object In JavaScript
To increment a value using dot notation, you would need to combine it with other operators or assignment expressions. Here’s an explanation of how to use dot notation along with other operators to increment a value in an object:
To increment a numerical value in an object using dot notation, you can retrieve the current value, perform the increment operation, and assign the updated value back to the property. Here’s an example:
var obj = {
count: 10
};
obj.count = obj.count + 1; // Incrementing using dot notation and addition operator
console.log(obj.count); // Output: 11
In the example above, we have an object obj
with a property called count
initially set to 10
. To increment the count
property, we use dot notation (obj.count
) to access its current value, add 1
to it, and then assign the updated value back to obj.count
. The resulting value is 11
.
You can also use compound assignment operators like +=
to achieve the same result more concisely:
obj.count += 1; // Incrementing using dot notation and += operator
console.log(obj.count); // Output: 11
In this case, obj.count += 1
is equivalent to obj.count = obj.count + 1
, incrementing the count
property by 1
.
Bracket Notation
Bracket notation is another way to access and modify properties of an object in JavaScript. It involves using square brackets ([]
) with the property name inside them. Here’s an explanation of how to use bracket notation to increment a value in an object:
To increment a numerical value in an object using bracket notation, you can retrieve the current value, perform the increment operation, and assign the updated value back to the property. Here’s an example:
var obj = {
count: 10
};
obj['count'] = obj['count'] + 1; // Incrementing using bracket notation and addition operator
console.log(obj.count); // Output: 11
In the example above, we have an object obj
with a property called count
initially set to 10
. To increment the count
property, we use bracket notation (obj['count']
) to access its current value, add 1
to it, and then assign the updated value back to obj['count']
. The resulting value is 11
.
Similarly, you can use compound assignment operators like +=
to achieve the same result more concisely:
obj['count'] += 1; // Incrementing using bracket notation and += operator
console.log(obj.count); // Output: 11
In this case, obj['count'] += 1
is equivalent to obj['count'] = obj['count'] + 1
, incrementing the count
property by 1
.
Bracket notation is especially useful when the property name needs to be dynamic or is stored in a variable. You can use a variable within the brackets to access or modify the property. For example:
var propertyName = 'count';
obj[propertyName] += 1; // Incrementing using bracket notation and a variable
console.log(obj.count); // Output: 11
In this case, the value of the propertyName
variable is used to access and increment the corresponding property.
Bracket notation provides flexibility for accessing and modifying object properties, especially when the property names are dynamic or contain special characters.
Nullish coalescing (??)
Nullish coalescing (??) is an operator introduced in JavaScript that provides a convenient way to handle null or undefined values. It allows you to provide a default value when encountering nullish values. Here’s an explanation of how to use nullish coalescing to increment a value in an object:
To increment a numerical value in an object using nullish coalescing, you can retrieve the current value, apply the nullish coalescing operator to provide a default value (if necessary), perform the increment operation, and assign the updated value back to the property. Here’s an example:
var obj = {
count: 10
};
obj.count = (obj.count ?? 0) + 1; // Incrementing using nullish coalescing and addition operator
console.log(obj.count); // Output: 11
In the example above, we have an object obj
with a property called count
initially set to 10
. To increment the count
property, we use nullish coalescing obj.count ?? 0
to check if the value of obj.count
is null or undefined. If it is nullish, the expression evaluates to 0
, which acts as the default value. Then, we add 1
to the result and assign the updated value back to obj.count
.
The nullish coalescing operator ensures that the increment operation occurs only when the value is not null or undefined, preventing any unwanted errors or undesired behavior.
Logical OR (||) Operator
The logical OR (||
) operator is a commonly used operator in JavaScript that can be leveraged to handle default values and perform operations conditionally. Here’s an explanation of how to use the logical OR operator to increment a value in an object:
To increment a numerical value in an object using the logical OR operator, you can retrieve the current value, apply the logical OR operator to provide a default value (if necessary), perform the increment operation, and assign the updated value back to the property. Here’s an example:
var obj = {
count: 10
};
obj.count = (obj.count || 0) + 1; // Incrementing using logical OR operator and addition operator
console.log(obj.count); // Output: 11
In the example above, we have an object obj
with a property called count
initially set to 10
. To increment the count
property, we use the logical OR operator obj.count || 0
to check if the value of obj.count
is a falsy value (e.g., null, undefined, 0). If it is falsy, the expression evaluates to 0
, which acts as the default value. Then, we add 1
to the result and assign the updated value back to obj.count
.
The logical OR operator ensures that the increment operation occurs only when the value is not falsy, preventing any unwanted errors or undesired behavior.
Conclusion – Increment A Value In An Object In JavaScript
In JavaScript, there are several approaches to increment a value in an object.
You can use dot notation or bracket notation to access and modify the property directly, then perform the increment operation. Alternatively, you can use compound assignment operators like +=
to increment the value more concisely. These methods require explicitly retrieving the current value, performing the increment, and assigning the updated value back to the property.
Additionally, you can leverage nullish coalescing (??
) or the logical OR (||
) operator to handle default values while incrementing. Nullish coalescing allows you to provide a default value when encountering null or undefined values, while the logical OR operator helps conditionally provide a fallback value when the property value is falsy.
Choosing the appropriate method depends on your specific use case and preferences. Consider the structure of your object, whether a default value is needed, and the desired behavior when handling nullish or falsy values.