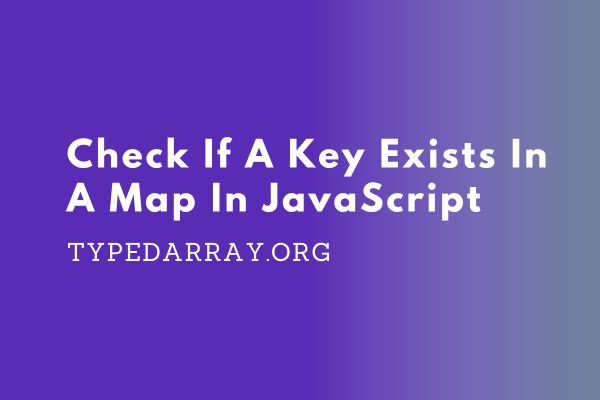
You can use several methods to check if a key exists in a map in JavaScript. Here are a few common ways:
- Using the
has()
method: Thehas()
method of theMap
object returns a boolean indicating whether the specified key exists in the map or not. - Using the
get()
method: Theget()
method of theMap
object returns the value associated with the specified key, orundefined
if the key does not exist. You can use this method to check if the returned value isundefined
. - Using the
keys()
method: Thekeys()
method of theMap
object returns an iterator of all the keys in the map. You can then use array methods likeincludes()
orindexOf()
to check if a specific key exists in the array of keys.
These are some of the common ways to check if a key exists in a map in JavaScript. Choose the method that best suits your needs based on the context and requirements of your code.
Check If A Key Exists In A Map In JavaScript
Let’s look at each of the above ways to check if a key exists on a map in JavaScript.
Using The has() Method To Check If A Key Exists In A Map In JavaScript
To check if a key exists in a Map
object specifically, you can use the has()
method, as mentioned earlier. Here’s an example:
const map = new Map();
map.set('key1', 'value1');
map.set('key2', 'value2');
console.log(map.has('key1')); // true
console.log(map.has('key3')); // false
The has()
method returns a boolean value indicating whether the specified key exists in the Map
object.
Using The get() Method
You can use the get()
method of the Map
object to indirectly check if a key exists. Here’s an example:
const map = new Map();
map.set('key1', 'value1');
map.set('key2', 'value2');
console.log(map.get('key1') !== undefined); // true
console.log(map.get('key3') !== undefined); // false
In this example, map.get('key1')
will return the corresponding value for 'key1'
, and checking if it’s !== undefined
allows you to determine if the key exists in the map. If the key is not present, map.get('key3')
will return undefined
, and the expression map.get('key3') !== undefined
will evaluate to false
.
Please note that this approach relies on the fact that undefined
is not a valid value for a key in a Map
. If you have keys with explicit undefined
values, this method may not work as intended. In such cases, it’s better to use the has()
method to directly check if a key exists in the Map
.
Using The keys() Method
If you want to check if a key exists in a Map
object using the keys()
method, you can convert the keys to an array and then use array methods like includes()
or indexOf()
to perform the check. Here’s an example:
const map = new Map();
map.set('key1', 'value1');
map.set('key2', 'value2');
console.log([...map.keys()].includes('key1')); // true
console.log([...map.keys()].includes('key3')); // false
In this example, map.keys()
returns an iterator of all the keys in the Map
. By spreading the iterator into an array using [...map.keys()]
, you can get an array of keys. Then, you can use the includes()
method to check if a specific key exists in the array of keys.
Alternatively, you can use the indexOf()
method to check the index of a specific key in the array of keys. If the key is found, indexOf()
will return a non-negative index. Otherwise, it will return -1.
const map = new Map();
map.set('key1', 'value1');
map.set('key2', 'value2');
console.log([...map.keys()].indexOf('key1') !== -1); // true
console.log([...map.keys()].indexOf('key3') !== -1); // false
Please note that converting the keys to an array using [...map.keys()]
creates a new array each time you perform the check. If you need to perform multiple key existence checks, it’s more efficient to store the keys in a separate array and reuse it for the checks.
Conclusion
In conclusion, when working with JavaScript maps, the most straightforward and reliable method to check if a key exists is by using the has()
method. This method directly checks if the specified key exists in the map and returns a boolean value indicating the result.
Alternatively, using the get()
method in combination with checking for undefined
can indirectly determine if a key exists by retrieving the value associated with the key. However, this approach may not be suitable if you have explicit undefined
values associated with keys.
Additionally, it’s worth mentioning that the keys()
method can be used to obtain an iterator of all the keys in a map. By converting this iterator into an array, you can utilize array methods like includes()
or indexOf()
to check for key existence. However, this approach creates a new array each time and might not be the most efficient if you need to perform multiple key existence checks.
To summarize, the has()
method is the recommended and straightforward approach to check if a key exists in a JavaScript map. It provides a clean and efficient way to determine whether a specific key is present in the map.