To get first n elements of an array in JavaScript, you can use any of these methods –
- Use Array.slice() method with 0 as the first argument and n as the second argument.
- Use Array.filter() method with a callback function that takes an element as argument and returns true if the index of the element is less than n.
- Use Array.findIndex() method with a callback function that takes an element as argument and returns true if the index of the element is equal to n-1.
- Use a for loop with a break statement inside the loop.
Let’s look at each of these methods in detail.
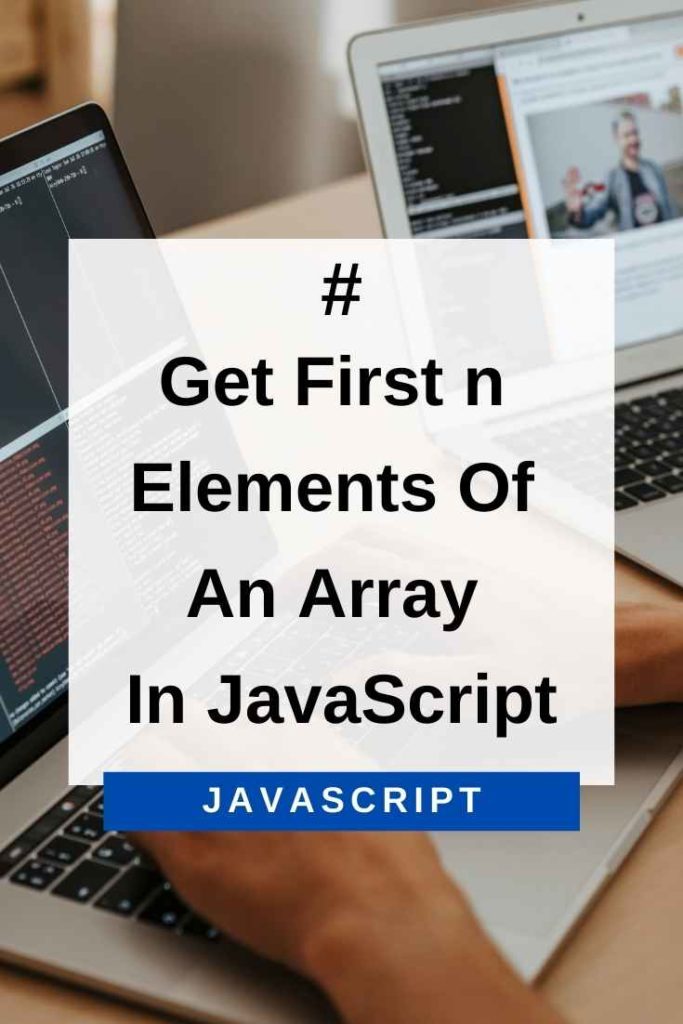
Array.slice() To Get First n Elements Of An Array In JavaScript
Use Array.slice() method with 0 as the first argument and n as the second argument
Array.slice() is a JavaScript array method that returns a shallow copy of a portion of an array into a new array object selected from start to end (end not included) where start and end represent the index of items in that array. The original array will not be modified.
If you omit the end parameter, slice() selects all elements from the start position to the end of the array.
Here’s an example to get first 3 elements of an array –
let fruits = [‘apple’, ‘banana’, ‘mango’, ‘orange’];
console.log(fruits.slice(0, 3)); // [‘apple’, ‘banana’, ‘mango’]
Array.filter() To Get First n Elements Of An Array In JavaScript
Use Array.filter() method with a callback function that takes an element as argument and returns true if the index of the element is less than n
Array.filter() is a JavaScript array method that creates a new array with all elements that pass the test implemented by the provided function.
If you provide a callback function as its argument, it will call the callback function once for each element in the array, and return a new array with only those elements for which the callback function returns a truthy value.
If you don’t provide a callback function, it will return a new array with all elements that pass the Boolean test.
Here’s an example to get first 3 elements of an array using Array.filter() method –
let fruits = [‘apple’, ‘banana’, ‘mango’, ‘orange’];
console.log(fruits.filter((fruit, index) => index < 3)); // [‘apple’, ‘banana’, ‘mango’]
For Loop To Get First n Elements Of An Array In JavaScript
Use a for loop with a break statement inside the loop.
A for loop is a type of loop that runs a specific number of times. It consists of three parts –
The initialization where you initialize the variable used in the loop.
The condition where you specify the condition to be checked before each iteration.
The increment where you increase or decrease the value of the variable used in the loop.
A break statement is used to exit a for loop prematurely.
Here’s an example to get first 3 elements of an array using for loop –
let fruits = [‘apple’, ‘banana’, ‘mango’, ‘orange’];
for (let i = 0; i < fruits.length; i++) {
if (i === 3) break;
console.log(fruits[i]);
}
Output
apple
banana
mango
Get First n Elements Of An Array In JavaScript – Summary
To get first n elements of an array in JavaScript, you can use any of these methods –
- Use Array.slice() method with 0 as the first argument and n as the second argument.
- Use Array.filter() method with a callback function that takes an element as argument and returns true if the index of the element is less than n.
- Use a for loop with a break statement inside the loop.