To get the index of an array element matching a condition in JavaScript, use the findIndex() method. This method takes a callback function as an argument and returns the index of the first element in the array that satisfies the condition.
If no element in the array satisfies the condition, then findIndex() returns -1.
Get The Index Of An Array Element Matching A Condition In JavaScript
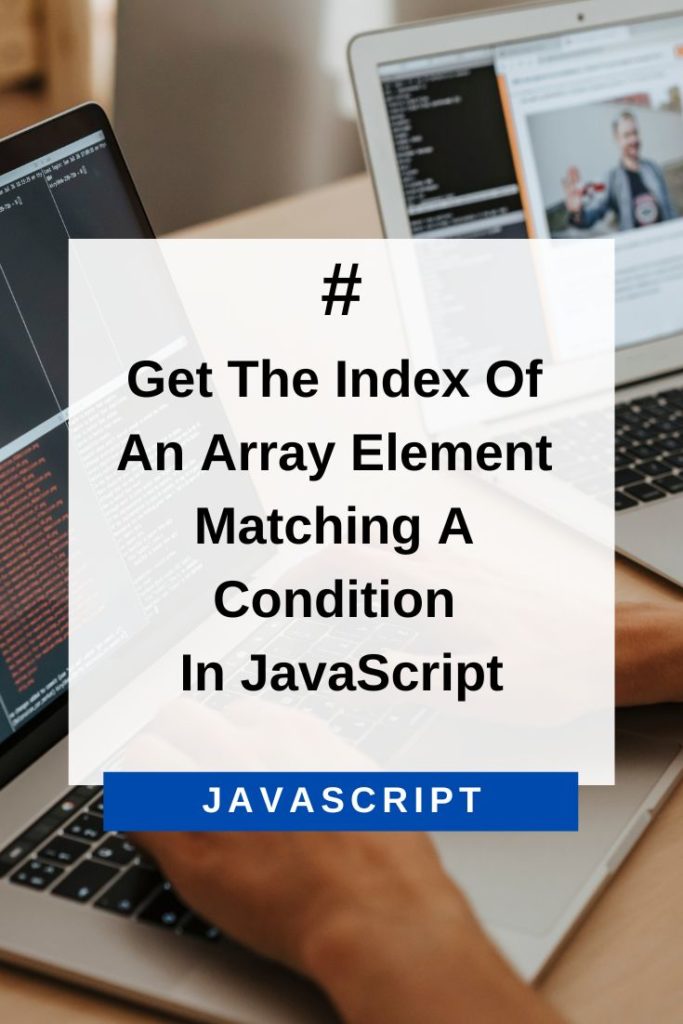
findIndex() To Get The Index Of An Array Element With Condition
Example:
const numbers = [-5, -4, -3, -2, -1, 0, 1, 2, 3, 4];
const firstNegative = numbers.findIndex(number => number < 0);
console.log(firstNegative); // Output: 0
const firstPositive = numbers.findIndex(number => number >= 0);
console.log(firstPositive); // Output: 5
In the first example, findIndex() returns the index of the first element in the array that satisfies the condition (i.e. the first negative number). In the second example, findIndex() returns the index of the first element in the array that satisfies the condition (i.e. the first positive number).
If no element in the array satisfies the condition, then findIndex() returns -1. For example:
const numbers = [-5, -4, -3, -2, -1, 0];
const firstPositive = numbers.findIndex(number => number > 0);
console.log(firstPositive ); // Output: -1
In this example, findIndex() returns -1 because there is no element in the array that satisfies the condition (i.e. there is no positive number).
You can also use a map() method with a filter() method to get the index of an array element matching a given condition. The map() method creates a new array with the results of calling a callback function on every element in the array. The filter() method creates a new array with all elements that pass the test implemented by the callback function.
map() And filter() To Get The Index Of An Array Element With Condition
Example:
const numbers = [-5, -4, -3, -2, -1, 0, 1, 2, 3, 4];
const allNegativeIndices = numbers.map((number, index) => number < 0 ? index : -1).filter(index => index !== -1);
console.log(allNegativeIndices); // [0,1,2,3,4]
In the first example, map() creates a new array with the indices of all elements if they are negative, and -1 otherwise. In the second example, filter() creates a new array with only those elements that pass the test (i.e. only the indices of negative numbers).
If no element in the array satisfies the condition, then the new array created by map() will be empty and filter() will return an empty array.
You can also use a for loop to get the index of an array element matching a given condition.
for Loop To Get The Index Of An Array Element With Condition
Example:
const numbers = [-5, -4, -3, -2, -1, 0, 1, 2, 3, 4];
let firstNegative;
for (let i = 0; i < numbers.length; i++) {
if (numbers[i]<0) {
firstNegative = i;
break;
}
}
console.log(firstNegative); // Output: 0
In the first example, the for loop breaks when it finds the first element in the array that satisfies the condition (i.e. the first negative number). The value of i is then logged to the console.
If no element in the array satisfies the condition, then the for loop will finish iterating over the entire array and firstNegative will be undefined.
Conclusion
There are several ways to get the index of an array element matching a condition in JavaScript. You can use the findIndex() method, the map() and filter() methods, or a for loop. Which method you choose will depend on your specific needs.