In JavaScript, there is a common error that you may run into when trying to add elements to a DOM node. This error is the “Cannot read property ‘appendChild’ of Null” error in JavaScript.
In this blog post, we will take a look at what this error means, and how you can fix it. We will also take a look at some of the most common causes for this error. So, if you are running into this error in your code, keep reading!
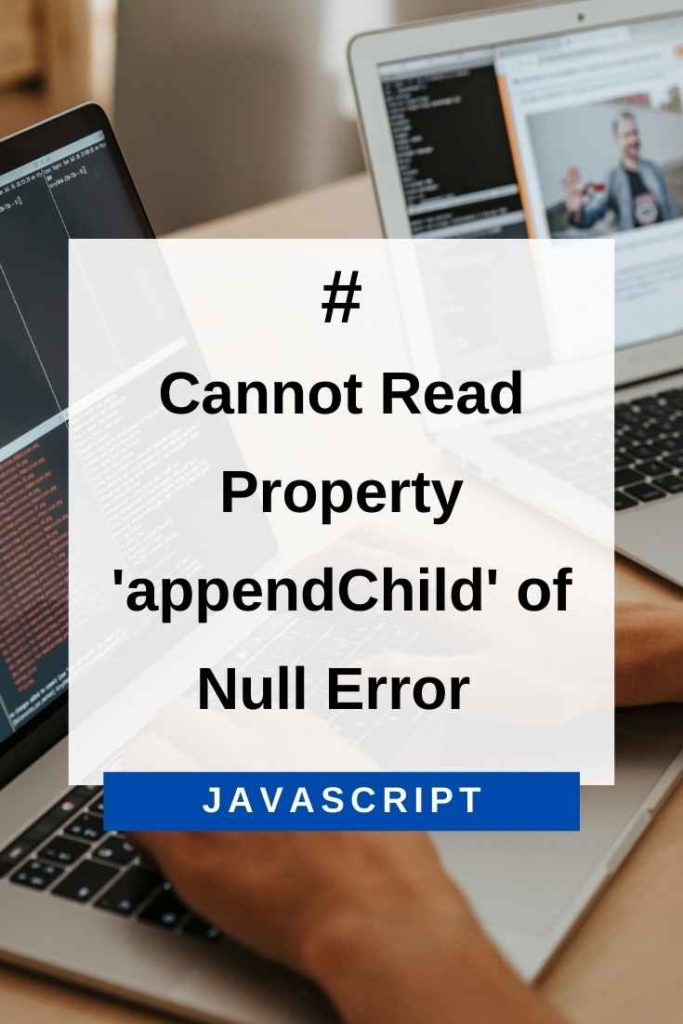
appendChild Method In JavaScript
The appendChild method is a method on the Node class. This method is used to add a node to the end of a child list for a given parent node. The appendChild method takes a single parameter, which is the node that you want to append to the parent.
For example, consider the following code:
var parent = document.getElementById("parent");
var child = document.createElement("div");
parent.appendChild(child);
In this code, we are first getting a reference to the parent node. Next, we are creating a new child node. Finally, we are using the appendChild method to append the child node to the parent.
However, if the parent node does not exist, then you will get the “Cannot read property ‘appendChild’ of Null” error.
This is because the appendChild method is trying to access the parent node, but the parent node does not exist. There are few more reasons why this error might occur, which we will explore next.
Cannot Read Property ‘appendChild’ Of Null – Reasons And Common Fixes
The “Cannot read property ‘appendChild’ of Null” error is usually caused by trying to add an element to a DOM node that does not exist. For example, if you write the code below –
document.getElementById("myDiv").appendChild(newElement);
But, “myDiv” does not exist in the DOM, you will get this error. This is because when you try to call the appendChild() method on a non-existent DOM node, it returns null.
You might have mistakenly declared the variable on which appendChild method is called, as null. For example, the code below would also lead to the same error.
var myDiv = null;
myDiv.appendChild(newElement);
This is because, when you try to call any method or property on a null variable in JavaScript, it will throw an error.
There are a few ways to fix this error. The first is to make sure that the node you are trying to add an element to actually exists in the DOM.
Another way to fix this error is to use a try/catch block around the code that is trying to append an element to the node. This will catch any errors that occur, and prevent them from being thrown.
Check this code for example – it will try to append an element to a node, but if the node does not exist, it will catch the error and prevent it from being thrown:
try {
myDiv.appendChild(someElement);
} catch (e) {
// Node does not exist, do nothing
}
You might also get the error if you place the script inside the html before the element you are trying to access, is declared. See the below code for example –
<script>
var myDiv = document.getElementById("myDiv");
myDiv.appendChild(newElement); //error
</script>
<div id="myDiv"></div>
The code above would cause the error because when the script runs, the element with id “myDiv” does not exist yet, as it is declared after the script.
To fix this, you should place the script at the end of the html document as shown below:
<div id="myDiv"></div>
<script>
var myDiv = document.getElementById("myDiv");
myDiv.appendChild(newElement); //no error
</script>
This way, when the script runs, the element will already exist in the DOM, and you will not get the error.
The “Cannot read property ‘appendChild’ of Null” error can also be caused by trying to append an element to a node that is not an element node. For example, if you have a text node, and you try to append an element to it, you will get this error. This is because only element nodes can have child nodes.
To fix this, you can use the node.parentNode property to get the parent node of the text node, and then append the element to that node. See the below code for an example:
var textNode = document.createTextNode("some text");
var parentNode = textNode.parentNode;
parentNode.appendChild(someElement);
A common cause for this error is forgetting to include a closing tag for an element. For example, if you have a div that contains a ul, and you forget to close the div, you will get this error when trying to add an element to the ul. This is because the div is considered to be a part of the ul, and not a separate node. To fix this, simply add a closing tag for the div.
Another common cause for this error is making a typo when trying to access a DOM node. For example, if you have a div with an id of “myDiv”, but you try to access it with the id “myDiv1”, you will get this error. This is because there is no node in the DOM with the id “myDiv1”. To fix this, make sure that you are using the correct id when trying to access a DOM node.
Check the below code for an example:
HTML:
<div id="myDiv"></div>
JavaScript:
var myDiv = document.getElementById("myDiv1"); //Wrong id!
myDiv.appendChild(someElement);
To fix this, simply use the correct id when trying to access the node. In this case, the correct code would be –
var myDiv = document.getElementById("myDiv");
Conclusion – Cannot Read Property appendChild Of Null Error In JavaScript
So, there you have it – a quick look at the “Cannot read property ‘appendChild’ of Null” error in JavaScript. We have looked at what this error means, and some of the common causes for it.
We have also seen how to fix this error by making sure that the node you are trying to append an element to actually exists in the DOM. Thanks for reading!