If you get a ‘replace is not a function’ error in JavaScript, it is because you have called the replace() method on a value that is not a string. To fix this, make sure you convert the value to a String using the toString() method before calling replace(). Alternatively, you can check the type of the value using the typeof operator to make sure it is a string before calling replace().
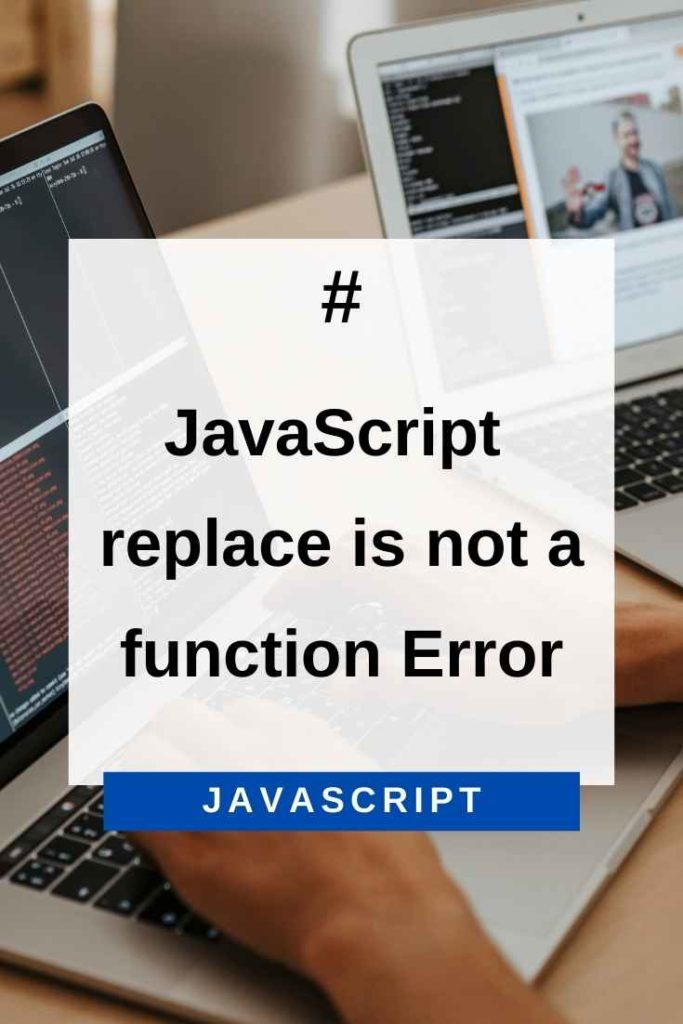
JavaScript ‘replace is not a function’ Error
If you’re working with strings in JavaScript, you may sometimes need to use the replace() method to modify or replace parts of the string. This method can be called on any string value, and it takes two arguments: the first argument is the substring that should be replaced, and the second argument is the replacement string.
However, if you try to call replace() on a value that is not a string, you will get an error saying “replace is not a function”. This is because the replace() method is only available on string values.
Here is an example of how the error occurs –
We try to call replace() on a number value:
var num = 10;
num.replace(/0/g, "5"); // "replace is not a function" error
To fix this, we can convert the num variable to a string using the toString() method before calling replace():
var replacedString = num.toString().replace(/0/g, "5");
console.log(replacedString); // "15"
Note that replace method doesn’t alter the original String. It just returns the replaced String.
Alternatively, we can use the typeof operator to check the type of the num variable before calling replace():
if (typeof num === "string") {
var replacedString = num.replace(/0/g, "5");
console.log(replacedString); //
} else {
console.log("num is not a string");
}
In the above code, we first check the type of num using typeof. If it is a string, we call replace() on it. Otherwise, we print an error message.
Let’s call this above code on num = 10;
if (typeof num === "string") {
var replacedString = num.replace(/0/g, "5");
console.log(replacedString); //
} else {
console.log("num is not a string");
}
Output:
“num is not a string”
As you can see from the output, we get an error because num is not a string.
What happens if we call the above code on num = “10” ?
if (typeof num === "string") {
var replacedString = num.replace(/0/g, "5");
console.log(replacedString);
} else {
console.log("num is not a string");
}
Output:
15
As you can see from the output, we don’t get an error because num is a string. We are able to successfully call replace() on it and replace 0 with 5.
Conclusion
As you can see, the “replace is not a function” error can occur in different situations.
To avoid this error, make sure you only call replace() on string values and that you convert any other type of value to a string before calling replace(). You can also use the typeof operator to check the type of a value before calling replace().