In this article, you are going to learn how you can calculate the time between two dates in JavaScript.
What Is A Date Object?
It is an in-built object in Javascript which is use to reference a certain point of time. By default, new Date() creates a Date object with the current date and time on the machine. We make the new object refer to our own timestamp by passing in the time as argument in the constructor function.
You can view the latest Mozilla Documentation Here.
How To Get The Time Difference?
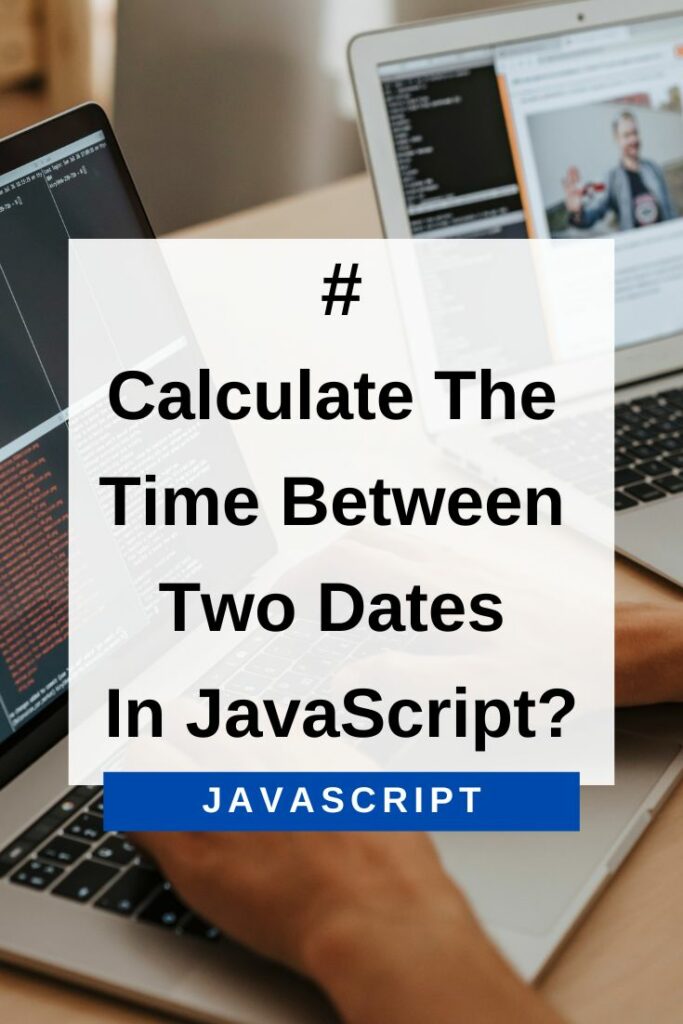
The steps we are going to follow :
- Create two date objects
- Subtract the date objects
- Divide the difference by the number of milliseconds in a day/week/month/year
- The result will be our desired output
π‘ Subtracting two dates gives the time difference in milliseconds.
What Is Unix Epoch?
In computing, Unix time is a system for describing a point in time. It is the number of seconds that have elapsed since the Unix epoch, which is 1st January 1970 at 00:00:00 GMT.
You can read more about it here.
The getTime Method
This method is defined on a date object by default and is used to get the milliseconds since Unix Epoch (1st January 1970 00:00:00 GMT).
π‘ getTime always returns the time difference between Unix Epoch and the current time in UTC, not your current time zone. Please keep this in mind.
How Do You Subtract Two Dates?
It is very straightforward to subtract two dates in Javascript. Simply use the β operator to subtract two dates like you subtract two numbers.
const date1 = new Date('2022-01-01');
// this is our smaller date
const date2 = new Date("2022-01-10");
// this is our larger date
const diff = date2 - date1;
console.log(diff)
// 777600000
Explanation: In this example, we create two date objects and showcase how you can calculate differences in dates just like in numbers.
How To Convert Milliseconds To The Desired Unit Of Time?
As you know, the difference is currently in milliseconds. We can convert it to any desired unit by dividing by the number of milliseconds per unit. So letβs calculate that :
Number of Milliseconds in a Week : Number of Days in a Week * Number of Hours in a Day * Number of minutes in an Hour * Number of seconds in a Minute * Number of milliseconds in a second
Number of Milliseconds in a Month : Number of Days in a Month * Number of Hours in a Day * Number of minutes in an Hour * Number of seconds in a Minute * Number of milliseconds in a second
Number of Milliseconds in a Day : Number of Hours in a Day * Number of minutes in an Hour * Number of seconds in a Minute * Number of milliseconds in a second
And likewise for other units of time.
How To Eliminate The Decimal Places?
We can use Math.floor, Math.ceil or Math.round.
Math.floor returns a number rounded down to the nearest integer
Math.round returns a rounded value, which means if the decimal part is equal to or greater than 0.5, add one to the largest integer. Otherwise, return the nearest integer
Math.ceil is returns a number rounded up to the nearest integer
// Math.round
Math.round(0.5) // returns 1
Math.round(1.1) // returns 1
Math.round(0.8) // returns 1
// Math ceil
Math.ceil(0.5) // returns 1
Math.ceil(1.1) // returns 2
Math.ceil(0.9) // returns 1
// Math.floor
Math.floor(0.5) // returns 0
Math.floor(1.1) // returns 1
Math.floor(0.8) // returns 0
π‘ You can use any of these according to your needs.
The Complete Code
Letβs assemble the parts to get the complete code β
const date1 = new Date('2022-01-01');
// this is our smaller date
const date2 = new Date("2022-01-10");
// this is our larger date
const diff = date2 - date1;
const milliseconds_in_a_week = 604800000
const milliseconds_in_a_day = 86400000
const milliseconds_in_a_month = 2592000000
const weeks = Math.round(diff / milliseconds_in_a_week );
const days = Math.round(diff / milliseconds_in_a_day );
const months = Math.round(diff / milliseconds_in_a_month );
console.log(weeks)
console.log(days)
console.log(months)
π‘ Remove the rounding function if you want decimal places too
The above code in functional format will be β
function getTimeDifference(date1,date2,unit){
let x = 0
if (unit == "month"){
x = 2592000000
} else if (unit == "week"){
x = 604800000
} else if (unit == "day"){
x = 86400000
}
// ... define other units according to your needs
return Math.floor(Math.abs(date2-date1) / x);
}
Summary
Here, you learned how to calculate the time between two dates in JavaScript in any unit, by using some simple mathematical operations on date objects. We also learned how you can use the various rounding functions.