If you get the “Cannot access ‘variable’ before initialization” error in JavaScript, it means that you’re trying to access a variable that hasn’t been declared yet. This can happen if you forget to declare a variable with the “var” keyword, or if you try to access a variable declared using let or const before it has been initialized.
Let’s discuss this error in detail below.
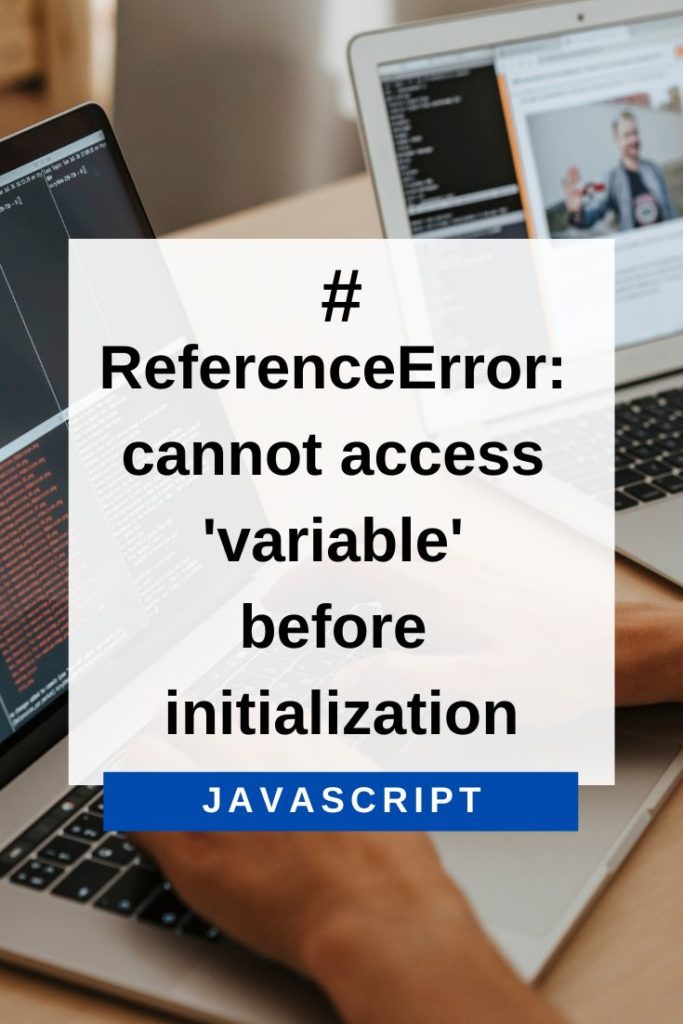
ReferenceError: cannot access ‘variable’ before initialization
The “cannot access ‘variable’ before initialization” error is a specific type of ReferenceError. This error is thrown when you try to access a variable that hasn’t been declared yet.
When you try to access a variable before it has been declared, you will get this error. This can happen if you are declaring a variable using let or const keywords, and try to access it before it has been declared.
Ex-
console.log(count); //ReferenceError: Cannot access count before initialization
let count = 0;
This is because when a variable is declared using let or const, it is available only in the scope in which it is defined.
If you use var instead of let or const , you will not get this error.
This is because variables declared with var are automatically hoisted to the top of the function, or global scope if they are not inside a function. This means that you can access them before they have been declared.
Hoisting is a process of moving variables and function declarations to the top of the scope before code execution.
In JavaScript, all var variables are automatically hoisted to the top of the function or global scope. This means that you can access them before they have been declared.
Here is an example –
console.log(myAge); //undefined
var myAge = 31;
In the above example, we are trying to access the “myAge” variable before it has been declared. This does not result in an error because var variables are hoisted.
However, you will get a different error if you try to access a let or const variable before it has been declared.
This is because variables declared with let or const are not hoisted. They are only available in the scope in which they are declared. This means that you cannot access them before they have been declared.
Here is an example of this –
console.log(myAge); //ReferenceError: cannot access myAge before initialization
let myAge = 31;
In the above example, we are trying to access the “myAge” variable before it has been declared. This results in a reference error because let variables are not hoisted.
The same is true for const variables.
Here is an example of this –
console.log(myAge); //ReferenceError: cannot access myAge before initialization
const myAge = 31;
In the above example, we are trying to access the “myAge” variable before it has been declared. This results in a reference error because const variables are not hoisted.
This is one of the key differences between var and let/const variables.
It is important to note that only the declaration of a var variable is hoisted to the top of the scope.
The assignment is not hoisted. This means that if you try to access a var variable before it has been declared and assigned, you will get an undefined error.
Here is an example of this –
console.log(myAge); //undefined
var myAge;
myAge = 31;
This is what it translates to behind the scenes –
var myAge;
console.log(myAge); //undefined
myAge = 31;
In the above example, we are trying to access the “myAge” variable before it has been declared and assigned. This results in an undefined error because only the declaration is hoisted, not the assignment.
Conclusion – cannot access ‘variable’ before initialization Error In JavaScript
In this article, we discussed the “cannot access ‘variable’ before initialization” error. This error is thrown when you try to access a variable that hasn’t been declared yet.
This can happen if you are declaring a variable using let or const keywords, and try to access it before it has been declared.
If you use var instead of let or const, you will not get this error, because variables declared using var are hoisted to the top of the scope.
This means that they can be accessed before they have been declared. The same is not true for variables declared using let and const.
It should be noted that only the declaration is hoisted, not the assignment. So, the variable holds the value undefined until it is declared and assigned a value.
Thank you for reading!