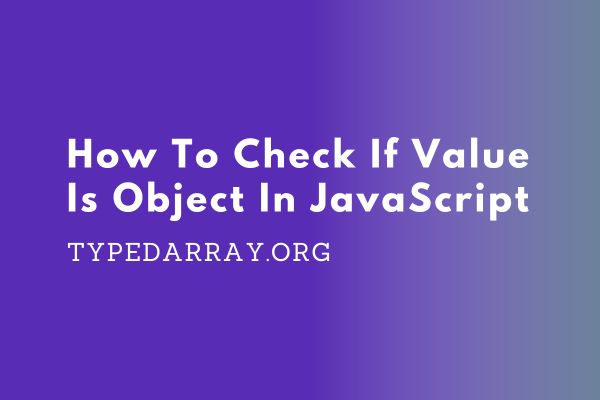
You can check if value is object in JavaScript using the typeof
operator and comparing it with the string “object”. However, this approach has some limitations because it treats certain types as objects that are not technically objects, such as null
and arrays.
Using typeof To Check If Value Is Object In JavaScript
Let’s create multiple variables of different types and we will then apply the `typeof` operator on each of them and print the result in the console.
const var1 = "hello";
const var2 = 2;
const var3 = [1, 2, 3];
const var4 = false;
const var5 = {};
const var6 = null;
console.log(typeof var1);
console.log(typeof var2);
console.log(typeof var3);
console.log(typeof var4);
console.log(typeof var5);
console.log(typeof var6);
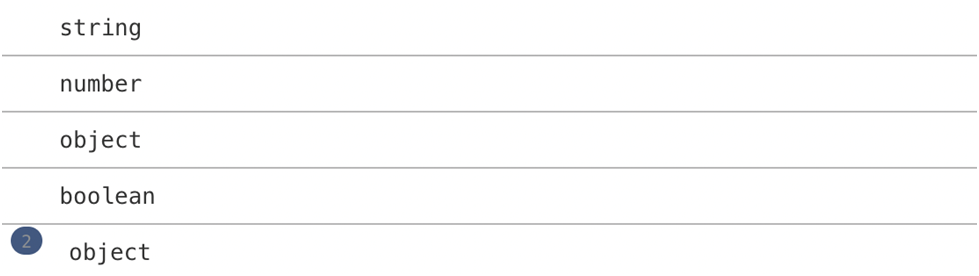
As we can see, we got the type of each of these variables. But an important thing to note here is that 3 of these variables are categorized of type object and those are `var3`, `var5`, and `var6`. What we should keep in mind here, is that in JavaScript, arrays and null values are defined as object types, and this can cause a lot of issues if we depend on the `typeof` operator directly to check for an object type.
So, we must do 2 steps before applying the `typeof` operator to check for objects and those are:
- Check that value is not `null`
- Check that value is not an array
Let’s create a function that takes a variable as an argument and checks if a value is an object or not –
const isValueObject = (value) => {
if (value !== null && !Array.isArray(value) && typeof value === "object")
console.log("Value is an object");
else console.log("Value is not an object");
};
If we call the following function on all the 6 variables we defined earlier –
isValueObject(var1);
isValueObject(var2);
isValueObject(var3);
isValueObject(var4);
isValueObject(var5);
isValueObject(var6);

As we can see, we got “Value is not an object” for the first 4 variables, and for the last one as well, and we only got one value that is matched to be an object which is `var5` in our case. So, we managed to make sure that we do not account for `null` values or arrays which was going to give us false results.
Using Object.prototype.toString() To Check If Value Is Object In JavaScript
Instead of using the typeof
operator, you can also use Object.prototype.toString()
to check if a value is an object in JavaScript. This method provides a more accurate way to determine if a value is an object and can handle edge cases like differentiating between arrays and other objects.
Here’s an example of how you can use Object.prototype.toString()
for object detection:
function isObject(value) {
return Object.prototype.toString.call(value) === '[object Object]';
}
// Examples:
console.log(isObject({})); // true
console.log(isObject([])); // false
console.log(isObject(null)); // false
console.log(isObject(42)); // false
console.log(isObject('hello')); // false
In this approach, Object.prototype.toString.call(value)
returns a string representation of the value’s internal [[Class]]
property. If the value is an object, it will return '[object Object]'
. For arrays, it will return '[object Array]'
. For null
, it will return '[object Null]'
. This method allows for more accurate identification of objects, making it a robust alternative to the typeof
approach.
Why You Should Not Use instanceof
Let’s delve into the reasons why using instanceof
to check if a value is an object in JavaScript is not recommended:
- Limited to Constructors:
instanceof
is primarily designed to work with constructor functions and their prototypes. It checks if an object is an instance of a particular constructor. This means that it is only useful for checking against specific constructor functions and may not cover other object types, such as plain objects created using object literals. - Fails for Objects from Different Contexts: In scenarios where multiple global contexts exist, such as in cross-frame environments or when using multiple iframes,
instanceof
might not behave as expected. Objects from different frames have separate constructor functions, which can lead to incorrect results when usinginstanceof
across frames. - Incorrect Results for Certain Types: While
instanceof
works fine for built-in types like arrays and dates, it might yield unexpected results for some types, like regular expressions. For example, checking if a regular expression is an instance ofRegExp
usinginstanceof
returnsfalse
. - Inheritance Confusion:
instanceof
doesn’t only check for the exact type but also considers any inherited types. This can be problematic in cases where an object’s prototype chain has been modified or where complex inheritance patterns are involved, leading to confusion when determining the actual type. - Custom Objects and Prototypes: When working with user-defined objects and prototypes,
instanceof
relies on the object’s prototype chain. If the prototype chain is altered or customized,instanceof
might produce incorrect results, leading to potential bugs in your code.
Given these limitations and potential pitfalls, it is generally better to use other methods like typeof
, Object.prototype.toString()
, or specific type-checking functions (e.g., Array.isArray()
) for more accurate and reliable object type checking in JavaScript. These alternative methods provide a more consistent way to determine if a value is an object without the constraints and issues associated with instanceof
.
Why You Should Not Use lodash.isObject
lodash.isObject
method is a part of the Lodash library, a popular utility library in JavaScript. While it has its uses, there are some considerations and limitations to be aware of when deciding whether to use it for checking if a value is an object:
- Ambiguous Definition of “Object”: The
lodash.isObject
method checks if the value is an object, but it includes not just plain objects but also other non-primitive types like arrays, functions, and dates. This broad definition might not be what you want if you specifically need to determine if a value is a plain JavaScript object created using object literals. - Array Handling: One of the significant issues with
lodash.isObject
is that it returnstrue
for arrays as well. While arrays are technically objects in JavaScript, they are a specialized type of object with indexed properties. If you need to distinguish between arrays and plain objects, this method might not be suitable. - Function Detection:
lodash.isObject
also returnstrue
for functions, which are objects in JavaScript. While this might be intended behavior in some cases, it might not be what you expect if you are only interested in identifying plain objects.
Due to these ambiguities and discrepancies, using lodash.isObject
for simple object type checks might not be the best approach. Instead, consider using more precise methods like typeof
, Object.prototype.toString()
, or specific type-checking functions like Array.isArray()
or custom checks if you have specific requirements.
Keep in mind that the Lodash library might evolve, and the behavior of its methods may change with newer versions. Always consult the official documentation and consider the specific use case to determine the most appropriate method for checking object types.
Conlcusion
In conclusion, when it comes to checking if a value is an object in JavaScript, it is essential to carefully consider the method you choose to ensure accuracy and consistency. While various approaches are available, each has its own advantages and limitations.
The typeof
operator provides a quick and easy way to identify objects but may yield false positives for certain types, such as arrays and null
. The Object.prototype.toString()
method offers a more precise and reliable solution, distinguishing between various object types, but its syntax can be slightly more verbose.
On the other hand, the instanceof
operator is not recommended for general object type checking, as it is primarily intended for constructor functions and has limitations with cross-frame environments and complex inheritance patterns.
As for the lodash.isObject
method, while it has its uses in certain scenarios, its broad definition of “object” may lead to unintended results, particularly with arrays, functions, and dates
. Consequently, it may not be the ideal choice for simple object type checks.
Ultimately, the choice of method depends on your specific use case and requirements. Whether it is for basic type checks or more complex scenarios involving inheritance and cross-frame environments, understanding the strengths and limitations of each method empowers you to make informed decisions for accurately detecting object types in JavaScript.
Always consider the nuances of the method you choose and strive for a clear and reliable approach that aligns with your particular needs.