To parse a JSON Array in JavaScript, pass the JSON Array string to the JSON.parse() method. This method parses the string and returns a JavaScript equivalent. If the JSON String is invalid, it will throw a SyntaxError.
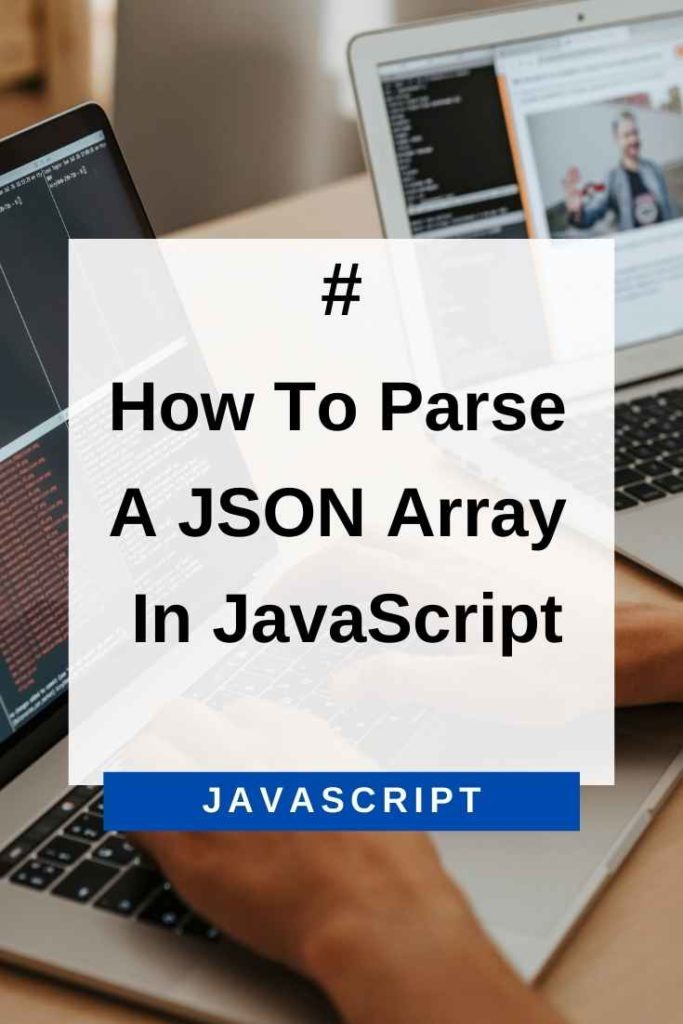
How To Parse A JSON Array In JavaScript
Use the JSON.parse() method to parse a JSON Array into a JavaScript equivalent.
The JSON.parse() method parses a string as JSON, optionally transforming the value produced by parse into a new value.
Syntax
JSON.parse(text, reviver)
Parameters
text
The string to parse as JSON. See the example below for details.
reviver
Optional. If a function, this prescribes how the value originally produced by parsing is transformed, before being returned.
Returns
The Object corresponding to the given JSON text, or null if the text is not valid JSON.
Exceptions
If the string to parse is not valid JSON, a SyntaxError exception is thrown.
Example
For example, the following string can be parsed into a JavaScript equivalent –
var jsonArrayString = ‘[{"name":"John","age":30},{"name":"Jane","age":25}]’;
var jsonArray = JSON.parse(jsonArrayString);
console.log(jsonArray);
Would output:
[{name: ‘John’, age: 30}, {name: ‘Jane’, age: 25}]
In the above code, we have a JSON Array string, and we are parsing it to a JavaScript array. We can then access the elements in the array, by their index.
For example, to get the name of the first person in the array, we would do –
var jsonArrayString = ‘[{"name":"John","age":30},{"name":"Jane","age":25}]’;
var jsonArray = JSON.parse(jsonArrayString);
console.log(jsonArray[0].name); // prints "John"
To get the name of the second person in the array, we would do –
var jsonArrayString = ‘[{"name":"John","age":30},{"name":"Jane","age":25}]’;
var jsonArray = JSON.parse(jsonArrayString);
console.log(jsonArray[1].name); // prints "Jane
If the JSON string is invalid, you will get a SyntaxError.
For example, the following string is not valid JSON –
var jsonArrayString = ‘[{"name":"John", "age":30},’;
var jsonArray = JSON.parse(jsonArrayString); // throws a SyntaxError
When you are dealing with JSON, it is important to remember that JSON is case-sensitive . This means that, for example, name and Name are two different keys.
Additionally, JSON requires double quotes (“) around strings. So, if you forget to put double quotes around a string, you will get a SyntaxError.
For example, the following is not valid JSON –
var jsonArrayString = ‘[{name:John,age:30},{name:Jane,age:25}]’;
var jsonArray = JSON.parse(jsonArrayString); // throws a SyntaxError
To fix these errors from being thrown, we can use the try/catch statement.
The try/catch statement will allow us to parse a string, and if there is an error thrown, it will be caught by the catch statement.
For example, we can use the try/catch statement to fix the previous errors –
var jsonArrayString = ‘[{"name":"John", "age":30},’;
try {
var jsonArray = JSON.parse(jsonArrayString);
} catch (error) {
console.log(error);
}
As you can see, using the try/catch statement can be helpful in debugging your code.
Keep in mind that the try/catch statement only works for synchronous code. This means that if you are using XMLHttpRequest or fetch , you will not be able to use the try/catch statement.
Instead, you should use the .then() method and handle any errors that are thrown in the .catch() method.
Always paste your code in a JSON validator, and check for any issues, before running your code. This will help you avoid any potential errors. You can use the replace() and replaceAll() methods to remove invalid characters from your JSON string.
For example, the following code will replace all single quotes (‘) with double quotes (“) –
var jsonArrayString = "{‘name’:’John’,’age’:’30’}";
jsonArrayString = jsonArrayString.replace(/’/g, ‘"‘);
console.log(jsonArrayString); // prints "name":"John","age":"30″
var jsonArray = JSON.parse(jsonArrayString);
console.log(jsonArray); // outputs { name: ‘John’, age: ’30’ }
You can also use a JSON Formatter to format and validate your JSON.
Conclusion
In this article, we learned about how to parse a JSON Array in javascript. We looked at how to use the JSON.parse() method and how to use the try/catch statement. Additionally, we also looked at how to use a JSON Formatter to format and validate your JSON.
I hope you found this article helpful. If you have any questions or comments, feel free to leave them below.