JavaScript is a versatile and powerful programming language that can be used to perform a wide range of tasks, including manipulating objects and data. One useful feature is the ability to easily get classname of an object in JavaScript.
In JavaScript, you can get the classname of an object using –
1. name property
2. instanceof operator
3. typeof operator
4. isPrototypeOf method
5. constructor property
Let’s have a look at each one of these methods in detail.
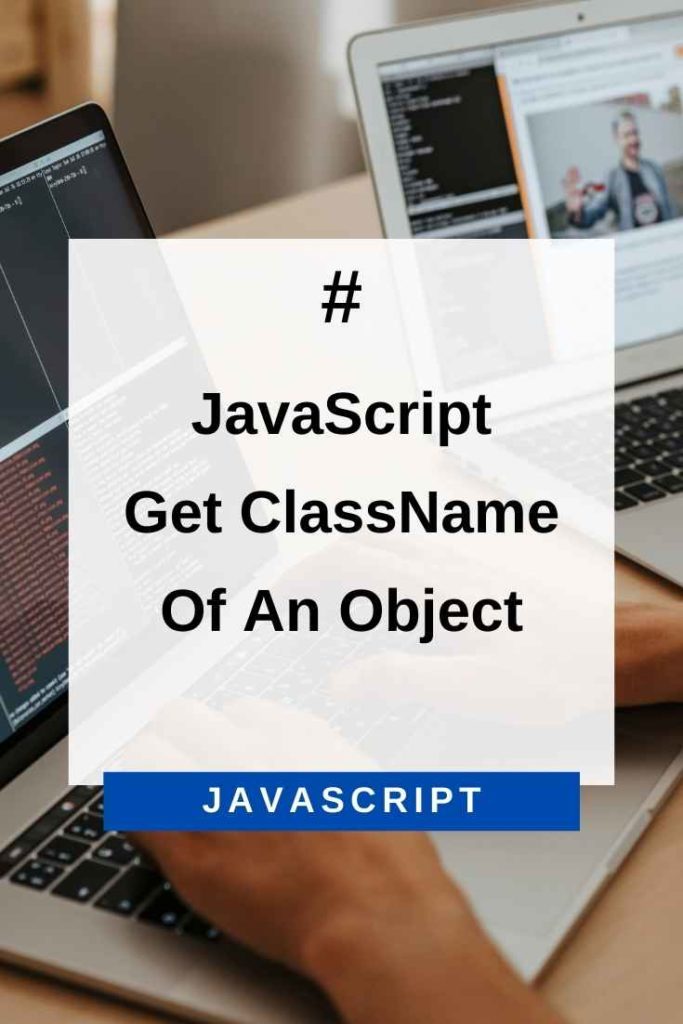
1. JavaScript name Property To Get Classname
The name property of the object’s constructor function gives you the classname.
For instance,
class Car {}
let car = new Car();
console.log("The name of the class is "+car.constructor.name); // outputs "The name of the class is Car"
console.log(Car.name); // outputs "Car"
2. JavaScript instanceof Operator To Get Classname
The instanceof operator is used to check whether an object belongs to a given constructor function or not.
For example,
class Car {}
let car = new Car();
console.log(car instanceof Car); // outputs true
3. JavaScript typeof Operator To Get ClassName
The typeof operator returns the type of the variable on which it is called.
It can be used to get the classname of an object as well.
class Car {}
let car = new Car();
console.log(typeof car); // outputs "object"
4. JavaScript isPrototypeOf() Method To Get ClassName
The isPrototypeOf() method is used to check whether an object exists in another object’s prototype chain or not.
class Car {}
let car = new Car();
console.log(Car.prototype.isPrototypeOf(car)); // outputs true
5. JavaScript constructor Property To Get ClassName
The constructor property of an object refers to the function that was used to create the object.
Using this property, you can get the classname of an object.
For example,
class Car {}
let car = new Car();
console.log(car.constructor); // outputs class Car {}
Thus, these were some of the methods that can be used to get the classname of an object in JavaScript. Which one you choose to use depends on your specific needs and requirements.
I hope this article helped you. Do share your feedback and queries in the comments section below.
Happy Coding! 🙂