You can replace single with double quotes in JavaScript using –
- replace() method
- replaceAll() method
Let’s see both of these methods one by one.
Replace Single With Double Quotes In JavaScript
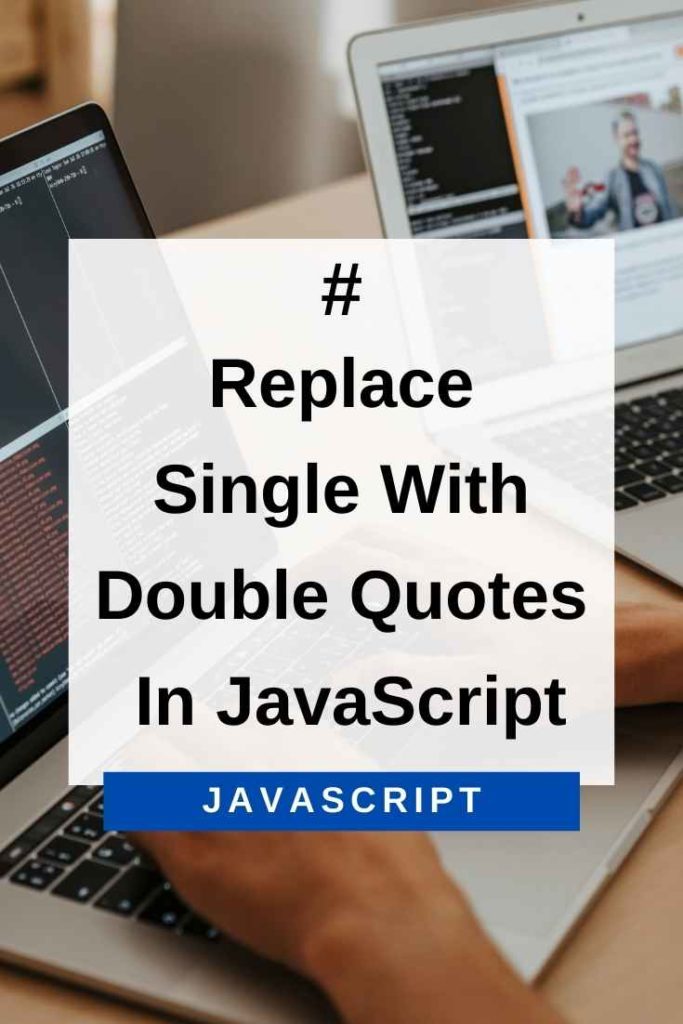
1. replace() method
The replace() method is used to replace a specified value with another value in a string.
This method does not change the original string, but it returns a new string with the replaced values.
Syntax:
str.replace(regexp|substr, newSubStr|function)
Parameters:
regexp (required): It is a RegExp that specifies the pattern of the string which is to be replaced.
newSubStr (optional): It is a string that replaces the matches found with the regexp parameter.
function (optional): It is a function that returns the new string.
This function is executed for each match found.
Return Value: It returns a new string with some or all matches of a pattern replaced by a replacement.
Example: This example replaces all the occurrences of single with double quotes in a string.
const str = "This is a string with ‘single’ quotes";
const newStr = str.replace(/’/g, ‘"‘);
console.log(newStr); // This is a string with "double" quotes
The / character is used as a delimiter here. The g character at the end of the regular expression means that the replace() method will replace all occurrences of single with double quotes.
2. replaceAll() method
The replaceAll() method is used to replace all the occurrences of a specified value with another value in a string.
This method does not change the original string, but it returns a new string with the replaced values.
Syntax:
str.replaceAll(string, replaceValue)
Parameters:
string (required): It is a string that specifies the pattern which is to be replaced.
replaceValue (optional): It is a string that replaces the matches found with the string parameter.
Return Value: It returns a new string with all of the occurrences of a specified value replaced by another value.
Example: This example replaces all the occurrences of single with double quotes in a string.
const str = "This is a string with ‘single’ quotes";
const newStr = str.replaceAll("‘", ‘"‘);
console.log(newStr); // This is a string with "double" quotes
In the above example, we have specified the string which is to be replaced i.e. ‘single’.
The method replaces all the occurrences of ‘single’ with double quotes.
The above code is quite confusing to read, as it uses alternating quotes. We can use backticks to make it more readable.
const str = "This is a string with ‘single’ quotes";
const newStr = str.replaceAll(`’`, `"`);
console.log(newStr); // This is a string with "double" quotes
The backticks (“) are used to specify template literals in JavaScript. They allow us to use quotes without having to escape them.
Conclusion
In this article, we looked at how to replace single with double quotes in JavaScript.
We saw two methods – replace() and replaceAll() that can be used for this purpose.
We also saw how backticks can be used to make our code more readable.