To push an object to an array in JavaScript, you can use the Array.push() method. The push() method adds one or more elements to the end of an array and returns the new length of the array. You can use the Array.unshift() method to push the object to the beginning of the array.
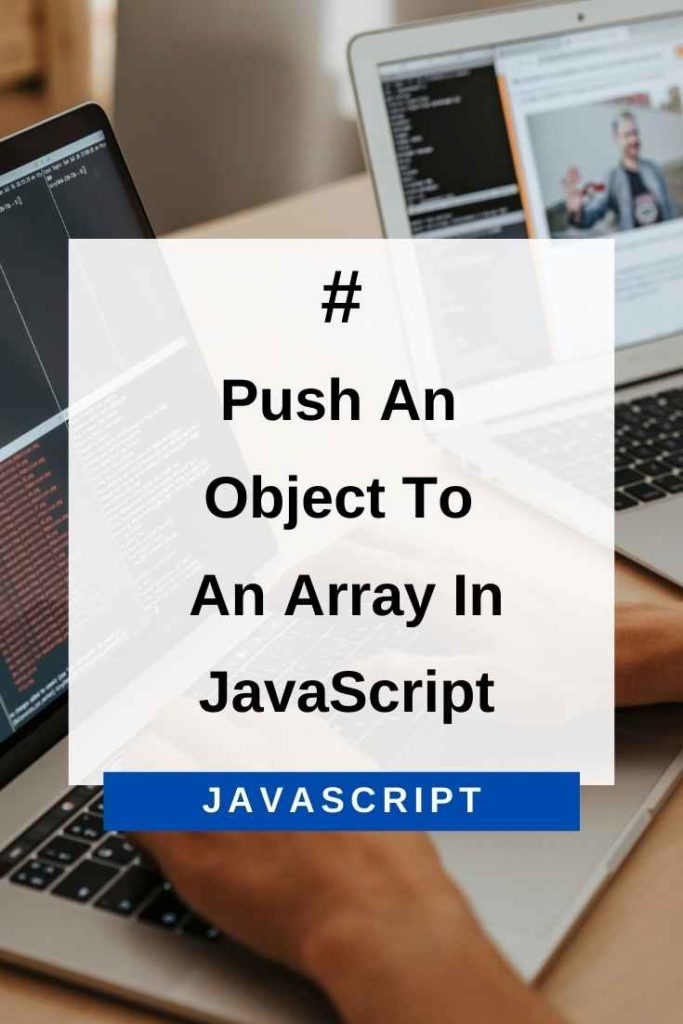
Push An Object To The End Of An Array In JavaScript
Here is an example of how to use the Array.push() method to push an object into an array:
var arr = [];
var obj = {name: "John", age: 30};
arr.push(obj);
console.log(arr);
// outputs:
// { name: "John", age: 30 }
As you can see from the example above, the Array.push() method can be used to add an object to an array. The object is added to the end of the array.
If you only have values, you need to create an object with those values and then push that object:
var arr = [];
var obj = {};
var name = "John";
var age = 30;
obj["name"] = name;
obj["age"] = age;
arr.push(obj);
console.log(arr);
// outputs:
// { name: "John", age: 30 }
You can add multiple objects to an array using the Array.push() method:
var arr = [];
var obj1 = {name: "John", age: 30};
var obj2 = {name: "Smith", age: 40};
arr.push(obj1);
arr.push(obj2);
console.log(arr);
// outputs:
// [{age: 30, name: "John"}, { age: 40, name: "Smith"}]
As you can see from the example above, the Array.push() method can be used to add multiple objects to an array. The objects are added to the end of the array.
Push An Object To The Beginning Of An Array In JavaScript
You can also use the Array.unshift() method to add one or more elements to the beginning of an array. The unshift() method returns the new length of the array.
Here is an example of how to use the Array.unshift() method to add an object to an array:
var arr = [];
var obj = {name: "John", age: 30};
arr.unshift(obj);
console.log(arr);
// outputs:
// { name: "John", age: 30 }
As you can see from the example above, the Array.unshift() method can be used to add an object to an array. The object is added to the beginning of the array.
If you had two objects, you could use the Array.unshift() method to add them both to the beginning of the array:
var arr = [];
var obj1 = {name: "John", age: 30};
var obj2 = {name: "Smith", age: 40};
arr.unshift(obj1);
arr.unshift(obj2);
console.log(arr);
// outputs:
// [{ age: 40, name: "Smith" }, { age: 30, name: "John"}]
As you can see from the example above, the Array.unshift() method can be used to add multiple objects to an array. The objects are added to the beginning of the array.
Conclusion – Push An Object To An Array In JavaScript
In this article, you learned how to use the Array.push() and Array.unshift() methods to add an object to an array in JavaScript. You also learned how to create an object with values and then push that object into an array. Finally, you learned how to add multiple objects to an array using the Array.push() and Array.unshift() methods.