To update all elements in an array in JavaScript, you can use any of these methods –
- Use the Array.forEach() method on the array, passing it a function and on each iteration, update the element of the array.
- Use the Array.map() method on the array, passing it a function and on each iteration, update the element of the array.
- Use a for loop to iterate through all elements of the array, updating each element in turn.
- You can even use the Array.reduce() method to update all elements of the array.
Let’s discuss each of these methods in detail below.
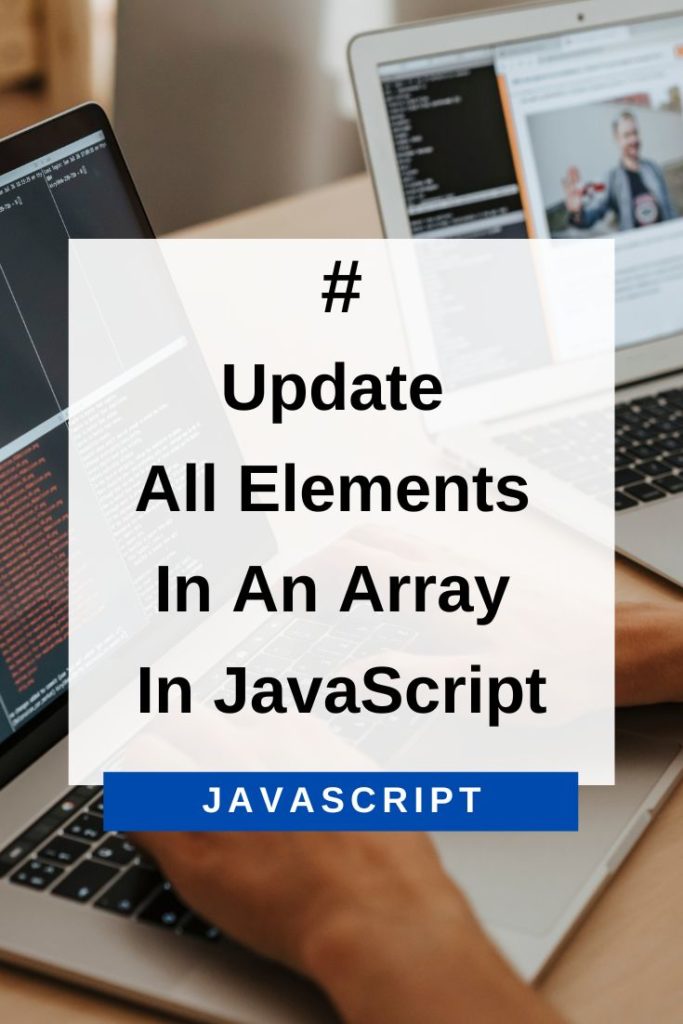
Use Array.forEach() Method To Update All Elements In An Array In JavaScript
The Array.forEach() method is used to iterate through each element of an array and perform a function on it. The first parameter of the forEach() method is the callback function that will be executed on each iteration. This function takes three parameters –
- the element currently being processed,
- the index of the element,
- the array being processed
,and it doesn’t return anything. The syntax of the forEach() method is as follows –
array.forEach(function(currentValue, index, arr), thisValue)
Now let’s see how we can use the forEach() method to update all elements of an array –
const arr = ['a', 'b', 'c'];
arr.forEach((element, index) => {
arr[index] = element.toUpperCase();
});
console.log(arr);
// Output – ['A', 'B', 'C']
In the above code, we have defined an array of three elements. We are using the forEach() method on this array and in the callback function, we are updating each element to its uppercase value. Finally, we are logging the updated array to the console.
Use Array.map() Method To Update All Elements In An Array In JavaScript
The Array.map() method is very similar to the forEach() method in the sense that it too iterates through each element of an array, applies a function on it and returns a new array with updated elements. The syntax of the map() method is as follows –
array.map(function(currentValue, index, arr), thisValue)
The map() method takes in a callback function as the first parameter and it returns a new array with updated elements. The callback function passed to map() is executed on each iteration and it too takes three parameters –
- the element currently being processed,
- the index of the element,
- the array being processed.
Now let’s see how we can use the map() method to update all elements of an array –
const arr = ['a', 'b', 'c'];
const updatedArr = arr.map((element, index) => {
return element.toUpperCase();
});
console.log(updatedArr);
// Output – ['A', 'B', 'C']
In the above code, we have first defined an array of three elements. We are using the map() method on this array and in the callback function, we are returning the uppercase value of each element.
The map() method returns a new array with updated elements and we are storing this array in the updatedArr variable. Finally, we are logging the updated array to the console.
Use A For Loop To Update All Elements In An Array In JavaScript
Another way to update all elements in an array in JavaScript is by using a for loop. A for loop iterates through each element of an array and we can use it to update the elements as well. The syntax of a for loop is as follows –
for (initialization; condition; increment) {
// Statements to be executed
}
Now let’s see how we can use a for loop to update all elements of an array –
const arr = ['a', 'b', 'c'];
for (let i = 0; i < arr.length; i++) {
arr[i] = arr[i].toUpperCase();
}
console.log(arr);
// Output – ['A', 'B', 'C']
In the above code, we have first defined an array of three elements. We are using a for loop to iterate through each element of this array. Inside the for loop, we are updating each element to its uppercase value.
Finally, we are logging the updated array to the console.
Use Array.reduce() Method To Update All Elements Of An Array
The Array.reduce() method is used to reduce an array of elements into a single value. The syntax of the reduce() method is as follows –
array.reduce(function(accumulator, currentValue, currentIndex, arr), initialValue)
The reduce() method takes in a callback function as the first parameter and it returns a single value. The callback function takes four parameters –
accumulator – accumulates the value returned by the callback function
currentValue – the current element being processed in the array
currentIndex – the index of the current element being processed in the array
arr – the original array
The second argument of the reduce method is an initial value.
Now let’s see how we can use the reduce() method to update all elements of an array in JavaScript –
const arr = ['a', 'b', 'c'];
const reducedArr = arr.reduce((acc, curr) => {
acc.push(curr.toUpperCase());
return acc;
}, []);
console.log(reducedArr);
// Output – ["A", "B", "C"]
In the above code, we have first defined an array of three elements. We are using the reduce() method on this array and in the callback function, we are adding each element to the accumulator array after converting it to uppercase.
The reduce() method returns a single value and we are storing this value in the reducedArr variable. Finally, we are logging the updated array to the console.
Conclusion
In this article, we saw how to update all elements of an array in JavaScript. We saw four different ways to achieve this –
- using the forEach() method,
- using the map() method,
- using a for loop, and
- using the reduce() method.
You can learn more about the map(), reduce(), and forEach() methods from MDN web docs.
I hope this article was helpful to you. If you have any questions, please feel free to post them in the comments section below and I will try my best to answer them.