To update an object’s property in array in JavaScript, you can use any of the following methods –
- Use Array.map() method to iterate over the array of objects and update a property for an object when found.
- Use Array.forEach() method to iterate over the array of objects and update a property for an object when found.
- Use a for() loop and update the property of an object when found.
Let’s have a look at each of these methods in detail.
Update An Object’s Property In Array In JavaScript
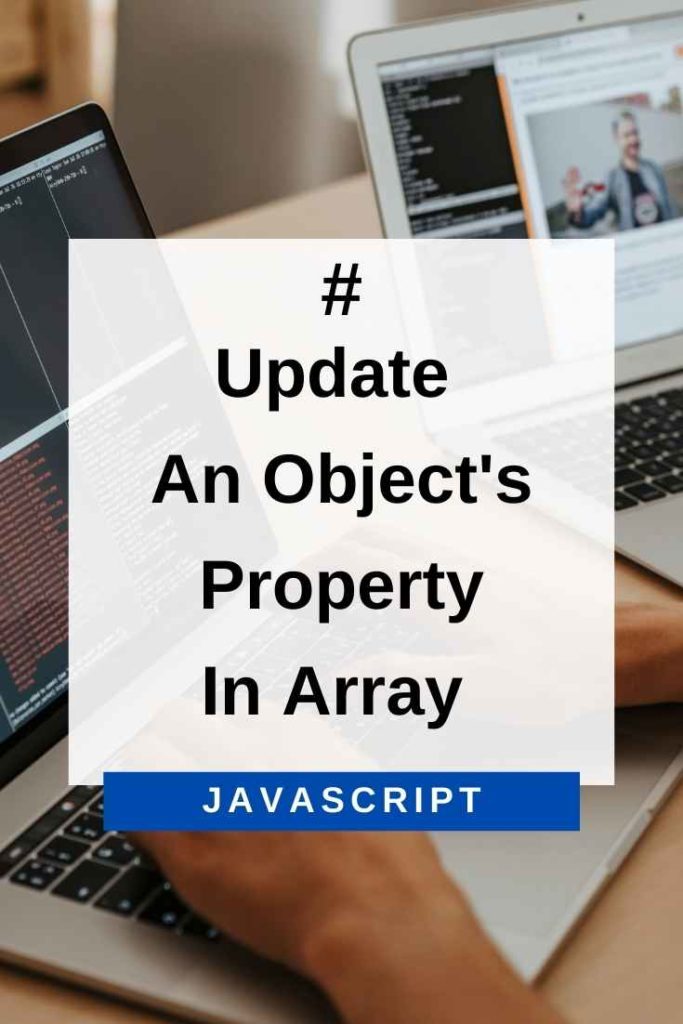
Use Array.map() Method
The map() method can be used to iterate over an array of objects and update a property for an object when found.
Here is an example –
let fruits = [{id:1, name:’apple’}, {id:2, name:’banana’}, {id:3, name:’orange’}];
const updatedFruits = fruits.map(fruit => {
if(fruit.id===1) {
fruit.name=’mango’;
return fruit;
}
return fruit;
});
console.log(updatedFruits);
This will give the output –
[{
id: 1,
name: “mango”
}, {
id: 2,
name: “banana”
}, {
id: 3,
name: “orange”
}]
In the above code, we have an array of objects – fruits. We have used the map() method to iterate over the array and update the property name for the object when its id is 1.
Use Array.forEach() Method
Another way to iterate over an array of objects and update a property for an object when found is to use the forEach() method.
Here is an example –
let fruits = [{id:1, name:’apple’}, {id:2, name:’banana’}, {id:3, name:’orange’}];
fruits.forEach(fruit => {
if(fruit.id===1) fruit.name="mango";
});
console.log(fruits);
This will give the output –
[{
id: 1,
name: “mango”
}, {
id: 2,
name: “banana”
}, {
id: 3,
name: “orange”
}]
In the above code, we have an array of objects – fruits. We have used the forEach() method to iterate over the array and update the property name for the object when its id is 1.
Use A for() Loop
Another way to update a property for an object when found in an array of objects is to use a for() loop.
Here is an example –
let fruits = [{id:1, name:’apple’}, {id:2, name:’banana’}, {id:3, name:’orange’}];
for(var i=0;i<fruits.length;i++) {
if(fruits[i].id === 1) {
fruits[i].name = "mango";
}
}
console.log(fruits);
This will give the output –
[{
id: 1,
name: “mango”
}, {
id: 2,
name: “banana”
}, {
id: 3,
name: “orange”
}]
In the above code, we have an array of objects – fruits. We have used a for() loop to iterate over the array and update the property name for the object when its id is 1.
Note that you can use the for loop to only update the first object who’s id is 1, in case there are multiple objects with id=1. The forEach() and map() method can be used when you have to update all the objects whose id is 1.
Here is an example –
let fruits = [{id:1, name:’apple’}, {id:2, name:’banana’}, {id:1, name:’orange’}];
for(var i=0;i<fruits.length;i++) {
if(fruits[i].id === 1) {
fruits[i].name = "mango";
break;
}
}
console.log(fruits);
In the above code, we have updated only the first object with id=1. If you want to update all the objects, use forEach() or map() method.
This will give the output –
[{
id: 1,
name: “mango”
}, {
id: 2,
name: “banana”
}, {
id: 1,
name: “orange”
}]
Conclusion
Thus, these are some of the ways in which you can update an object’s property in array in JavaScript. You can choose any of these methods depending on your requirement.
I hope this was helpful. Thanks for reading!