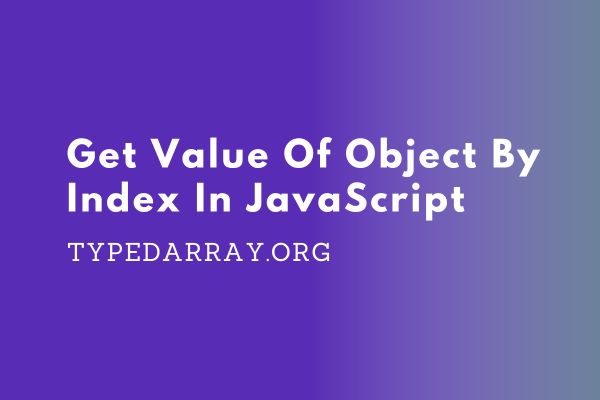
In this article, we will cover how to get value of object by index in JavaScript. To achieve this, we will use the following methods –
- Using Object.values()
- Using Object.keys()
- Using Object.entries()
- Using a for…in Loop
Get Value Of Object By Index In JavaScript Using Object.values()
Let’s start by creating an object and initializing it with some values –
const obj = {
key1: "value1",
key2: "value2"
};
Then we need to store the object’s values in an array using the `Object.values()` method –
const objectValues = Object.values(obj);
Let’s print `objectValues` on the console and see what we have –
console.log(objectValues);

As we see, we got an array with 2 values which are the values we defined inside `obj`.
So, now if we want to access the first value in our object which is `value1`, we can do this by accessing the first element in `objectValues` arrays which is at index `0` –
console.log(objectValues[0]);
We get `value1` as output which corresponds to the first value inside `obj`.
We can only access values that are inbounds of our array, so if our object has 2 values then the array size is 2, so we cannot access the third element in the array which is of index `2` as this will return undefined.
console.log(objectValues[2]);

Get Value Of Object By Index In JavaScript Using Object.keys()
Another approach is to use the `Object.keys()` method to get an array of the object keys and then get the key at the specified index and access the object by the key to get the corresponding value –
const objectKeys = Object.keys(obj);
Now we have an array with the object keys. We will then access the object passing it the key at the specified index using the bracket notation `obj[key[index]]` –
console.log(obj[objectKeys[0]]);
If we check the console, we will see that we got the value that corresponds to the key of index `0` which is the first value in our object.

We can wrap our logic inside a function block so that it can be applied on any object and specifying any index. We will be using the first approach which is using the `Object.values()` method. The function will take 2 arguments, the object we need to find a value inside, and the index of the value we need to find –
const getValueByIndex = (object, index) => {
return Object.values(object)[index];
};
Now let’s call our function passing in our object and index `0` to find the first object and print our output in the console –
console.log(getValueByIndex(obj, 0));

As we can see, we managed to get the value that matches the index we specified, and using this function, we can pass in another object and specify another index to search for the corresponding value.
Using Object.entries()
You can use Object.entries()
to get the value of an object by index. Here’s an example:
const obj = {
key1: 'value1',
key2: 'value2',
key3: 'value3'
};
const entries = Object.entries(obj);
const index = 1; // Index of the desired value
if (index >= 0 && index < entries.length) {
const desiredValue = entries[index][1];
console.log(desiredValue); // Output: 'value2'
} else {
console.log('Invalid index');
}
In the code above, Object.entries(obj)
returns an array of key-value pairs from the object obj
. You can then access the desired value using the index. The [index]
selects the key-value pair at the specified index, and [1]
accesses the value from that pair.
Using A for…in Loop
Here’s an example:
const obj = {
key1: 'value1',
key2: 'value2',
key3: 'value3'
};
const index = 1; // Index of the desired value
let currentIndex = 0;
let desiredValue;
for (const key in obj) {
if (obj.hasOwnProperty(key)) {
if (currentIndex === index) {
desiredValue = obj[key];
break;
}
currentIndex++;
}
}
if (desiredValue) {
console.log(desiredValue); // Output: 'value2'
} else {
console.log('Invalid index');
}
In the code above, the for...in
loop iterates over each enumerable property of the object obj
. By using the obj.hasOwnProperty(key)
check, we ensure that only the object’s own properties (not inherited ones) are considered.
Within the loop, we compare the currentIndex
with the desired index
. If they match, we assign the corresponding value to the desiredValue
variable and break out of the loop. If the loop finishes without finding a match, we output an “Invalid index” message.