If you are a beginner to JavaScript, you might be wondering – is JavaScript compiled or interpreted. The answer is both, depending on how you use it.
In this article, I will shed some light on what a compiled or interpreted language is, and whether JavaScript is either one.
So, to answer the question – is JavaScript interpreted or compiled? The answer is both, depending on the implementation. In general, it is considered an interpreted language. But, more recent versions are compiled, at least partially. This compilation happens dynamically and at runtime, so you don’t need to worry about it as a programmer.
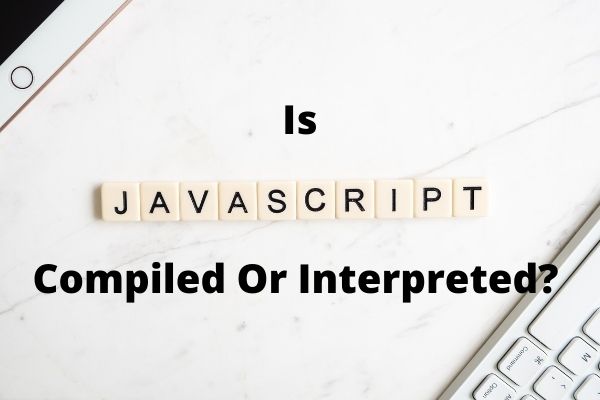
Compiled vs Interpreted Languages
A compiled language is one where the code you write is converted into machine code, before it is run. This machine code is then run directly by the processor, without the need for any further interpretation.
An interpreted language is one where the code you write is converted into an intermediate form, which is then run by an interpreter. The interpreter reads this intermediate code and executes it, line by line.
Let’s see this through a real-life example.
Say you want to bake a cake. The compiled language would be like having a recipe book with a list of ingredients and instructions on how to bake the cake. It will then add all the ingredients at once, mix them together and bake the cake.
In contrast, an interpreted language would be more like adding the ingredients one by one, as per the instructions given.
So, Which Is Better?
Well, it depends on who you ask. Some people argue that compiled languages are better because they are faster. This is because the machine code is already ready to be run and there is no need for interpretation.
Others argue that interpreted languages are better because they are more flexible. This is because you can change the code on the fly and see the results immediately. There is no need to recompile the code every time you make a change.
Is JavaScript Compiled Or Interpreted?
For me, the term “interpreted” or “compiled” is a touch of controversy. It’s a design choice rather than part of the language standard. If you want to discuss compiled or interpreted JavaScript in terms of a real implementation of the language specification, do so only if it is relevant.
In general, JavaScript is considered a dynamic, interpreted language. But, is it truly an interpreted language? Earlier versions of JavaScript were interpreted, but more recent versions are JIT compiled.
Exactly when the code is compiled to machine code depends on the specific implementation. In the recent version of V8, it starts by interpreting the code. If a piece of code is run multiple times, it will compile that code to machine code and optimize it for faster execution.
Why does this matter? It doesn’t really, but it’s interesting to know how JavaScript works under the hood.
This explains why modern JavaScript engines are so fast. There is no way JavaScript engine can compete with other implementations of JavaScript if it truly is interpreted.
V8 used to compile the code to machine code all at once, but this isn’t very efficient. The new approach of compiling only the code that is actually executed multiple times makes V8 much faster.
Some people argue that since you can see the JavaScript code in the browser, it is an interpreted language. But, the truth is that you can see the code because it is compiled only before getting executed.
WebAssembly is a new JavaScript feature that compiles AOT code (from languages other than JavaScript, such as C) into a more efficient format. In those situations, you would not be able to view the original source code.
Another feature that prevents you from seeing the source code is a binary AST in development. It would load quickly and begin executing right away.
There are a few more use cases that justify that modern JavaScript is compiled.
Hoisting
Consider the following example –
console.log(foo()); // Output: 10
function foo() {
var x = 10;
return x;
}
In the above example, despite calling the function foo before it is declared, we are still able to get output. This is because of something called hoisting.
In JavaScript, functions and variables are hoisted to the top of their scope before execution. So, even though the function foo is declared after it is called, due to hoisting, it is still executed.
But why does this happen?
It wouldn’t be possible if the code was not compiled before being executed. The compiler automatically moves the function declaration to the top, and that is why we don’t get an error.
Error Handling
Consider the following example –
console.log("hello");
oops oops;
We get the following error on running the code –
oops oops;
^^^^
SyntaxError: Unexpected identifier
In theory, if JavaScript was an interpreted language, the hello statement would have been printed before the error occurred. This is because the code would have been interpreted line by line.
But that’s not what happens. The browser halts the execution as soon as it encounters an error and doesn’t execute any further code.
This is again due to compilation. The code is compiled before being executed, and any errors are caught during compilation itself.
This enables us to fix errors quickly and efficiently, without having to worry about the rest of our code getting affected.
Thus, we can say that JavaScript is a compiled language, despite popular belief.
When the code is run in modern browsers, it is first parsed by a JavaScript engine. This parsing step produces an intermediate representation of the code. The engine then executes the code directly from this intermediate representation.
Let’s understand this process in detail.
JavaScript Program Flow
1. Tokenization
Tokenization is the process of breaking up a string of code into smaller tokens. Each token represents a single element from the code, such as a keyword, an identifier, or a punctuation character.
2. Parsing
Parsing is the process of taking a stream of tokens and converting it into a tree-like structure, known as a syntax tree. The nodes of this tree represent the different elements of the code, such as statements, declarations, and expressions.
3. Code Generation
Code generation is the process of taking a syntax tree and converting it into executable code. This code is typically in the form of bytecode or machine code.
Bytecode is a low-level code that can be interpreted by a virtual machine or runtime environment. Machine code is native code that can be executed directly by the processor.
4. Execution
Finally, the generated code is executed line by line by the JavaScript engine.
So, we can see that modern JavaScript engines compile the code before executing it. However, this compilation step is hidden from the programmer. All you need to do is write the code and run it in the browser.
The Just-In-Time (JIT) Compiler
So, if current versions of JavaScript are compiled, what does that mean for interpretation?
Well, most JavaScript engines nowadays use a technique called Just-In-Time (JIT) compilation. This means that the code is not actually compiled until it needs to be executed.
For example, if you have a loop that runs a thousand times, the JIT compiler will compile the code just before the loop starts running. This compiled code will then be executed directly by the processor, without the need for interpretation.
JIT compilation means that the parsing and compilation steps are done at the same time, and that the code is only compiled when it needs to be run.
Because of this, JavaScript can be both interpreted and compiled, depending on how you use it.
So, JavaScript cannot be considered a compiled language in the traditional sense because the code is not immediately converted to byte code or machine code.
However, it can be considered a compiled language because the code is eventually converted to an intermediate representation which is then executed.
This makes JavaScript a very fast and efficient language, despite its popularity as a scripting language.
JavaScript On The Web
When used on a web page, JavaScript code is interpreted by the browser’s JavaScript engine. The engine converts the code into an intermediate form, which it then executes.
This means that, when used on a web page, JavaScript is an interpreted language.
However, there are also ways to use JavaScript outside of a web page, in which case it can be either compiled or interpreted.
JavaScript In Node.js
Node.js is a platform for running JavaScript code outside of a web browser. It includes a runtime environment and a library of modules.
Code written for Node.js is typically either compiled to machine code using a Just-In-Time (JIT) compiler, or interpreted by the Node.js runtime.
So, when used with Node.js, JavaScript can be either compiled or interpreted.
JavaScript In V8
V8 is a JavaScript engine used in Google Chrome and Node.js. It compiles JavaScript code to machine code using a JIT compiler when certain functions are executed repeatedly.
So, when used with V8, JavaScript can be either interpreted or compiled.
JavaScript In SpiderMonkey
SpiderMonkey is a JavaScript engine used in Mozilla Firefox. It contains an interpreter and a JIT compiler.
When used with SpiderMonkey, JavaScript can be either interpreted or compiled.
Advantages Of JavaScript As A Compiled Language
One of the main advantages of JavaScript as a compiled language is that JavaScript code can be run more quickly.
Second, it makes your code more secure, because the source code is not visible to people who might want to exploit it.
Finally, it means that you can use JavaScript to create desktop applications and server-side applications.
All of these factors make JavaScript an attractive choice for web developers who want to create robust and high-performance web applications.
Advantages Of JavaScript As An Interpreted Language
The main advantage of using JavaScript as an interpreted language is that it doesn’t need to be compiled in order to run. This makes it very versatile and easy to work with, as there’s no need to set up a complicated build process.
Interpreted languages are also generally more portable than compiled languages, as they can be run on any system that has an interpreter.
JavaScript is also a very forgiving language, and will try to do its best to execute code even if it isn’t syntactically correct. This can be both a good and a bad thing, as it can make debugging code more difficult, but it also makes it possible to write “quick and dirty” code that will still run correctly.
Overall, the advantages of being an interpreted language make JavaScript a great choice for rapid prototyping and development.
Related Post: Is JavaScript An Object Oriented Programming Language?
Conclusion – Is JavaScript Compiled Or Interpreted?
JavaScript can be either a compiled or interpreted language, depending on how it is used. When used on a web page, it is interpreted by the browser’s JavaScript engine. When used with Node.js or V8, it can be either compiled or interpreted.
I hope this article has helped to clear up any confusion you might have had about whether JavaScript is a compiled or interpreted language. Thanks for reading!