To make Array.indexOf() case insensitive in JavaScript, use the Array.findIndex() method with a function that performs a case-insensitive comparison.
Make Array.indexOf() Case Insensitive In JavaScript
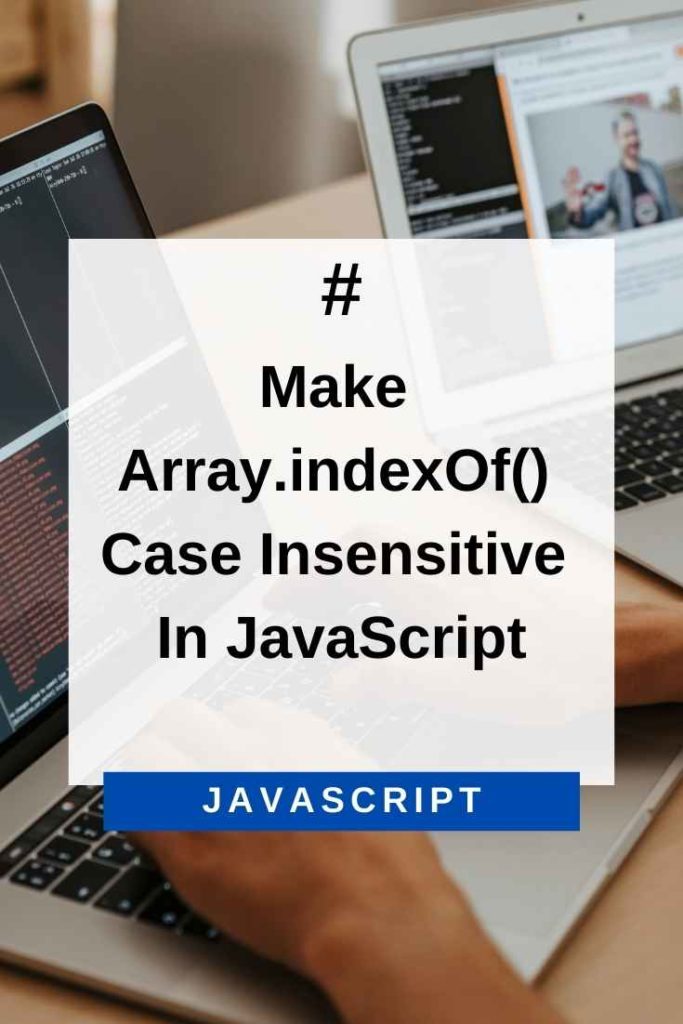
For example, given the following array:
var arr = ["a", "b", "c"];
You can use Array.findIndex() to find the index of an element case-insensitively:
var idx = arr.findIndex(function(el) { return el.toUpperCase() === "B"; });
console.log(idx); // 1
If the element is not found, Array.findIndex() returns -1:
var arr = ["a", "b", "c"];
var idx = arr.findIndex(function(el) { return el.toUpperCase() === "D"; });
console.log(idx); // -1
You can use a conditional statement to check if an element exists in an array case-insensitively:
var arr = ["a", "b", "c"];
var idx = arr.findIndex(function(el) { return el.toUpperCase() === "B"; });
if (idx > -1) {
console.log("element exists at index " + idx);
} else {
console.log("element does not exist in array");
}
This method is supported in all major browsers except Internet Explorer 11 and earlier.
Array.prototype.findIndex() is an improvement over the traditional for loop because it:
- stops searching once it finds the first element that meets the condition
- doesn’t require a break statement
- works with all types of arrays, including typed arrays
- is available in all major browsers except Internet Explorer 11 and earlier
Note that the function passed to Array.findIndex() must perform a case-insensitive comparison, as the default comparison is case-sensitive.
If you need to support older browsers, you can polyfill or babel.