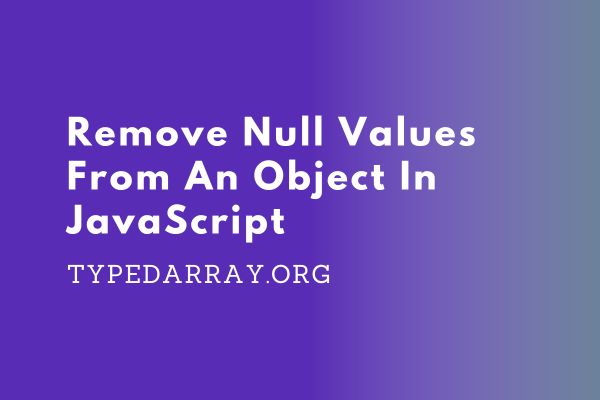
In JavaScript programming, objects are widely used to store and manipulate data. However, it’s not uncommon to encounter null values within objects, which can sometimes cause issues when working with the data. Null values represent the absence of a value or the intentional assignment of no value to a particular property within an object.
To ensure the integrity and consistency of data, it is often necessary to remove null values from an object. This process involves traversing the object, identifying properties with null values, and eliminating or modifying them according to the desired outcome. By removing null values, you can enhance the reliability and efficiency of your code, ensuring that only valid and meaningful data is processed.
In this guide, we will explore various approaches to remove null values from an object in JavaScript. We will cover both simple objects and nested objects, providing step-by-step explanations and code examples to illustrate the process. Whether you are a beginner or an experienced JavaScript developer, this guide will equip you with the knowledge and techniques needed to handle null values within objects effectively.
So, let’s dive in and learn how to remove null values from objects in JavaScript!
Remove Null Values From An Object In JavaScript
Here are several approaches you can use to remove null values from an object in JavaScript:
- Iterating and Filtering:
- Use
Object.keys()
orObject.entries()
to iterate over the object properties. - Use the
filter()
method to remove properties with null values.
- Use
- Iterating and Deleting:
- Use
for...in
loop to iterate over the object properties. - Use the
delete
keyword to remove properties with null values.
- Use
- Creating a New Object:
- Iterate over the object properties and create a new object excluding null values.
- Recursively Removing Nulls:
- For objects with nested properties, recursively traverse the object and remove null values at each level.
- Using Libraries:
- Utilize libraries like Lodash or Underscore.js, which provide convenient methods (e.g.,
_.omitBy()
) to remove null values from objects.
- Utilize libraries like Lodash or Underscore.js, which provide convenient methods (e.g.,
Remember to choose the approach that best suits your requirements and coding style. Each method has its advantages and considerations depending on the complexity of your object structure and performance requirements.
Feel free to explore these approaches in more detail and choose the one that fits your specific use case.
Iterating and Filtering
One of the ways to remove null values from an object in JavaScript is by iterating over the object properties and filtering out the properties with null values. Here’s an example of how you can do this:
const obj = {
name: 'John',
age: null,
occupation: 'Developer',
address: null,
};
const filteredObj = Object.keys(obj).filter(key => obj[key] !== null)
.reduce((acc, key) => {
acc[key] = obj[key];
return acc;
}, {});
console.log(filteredObj);
In this example, we start by declaring an object called obj
with some properties that contain null values. We then use Object.keys(obj)
to get an array of the object’s keys.
We apply the filter()
method on this array, using a callback function that checks if the value of each property (obj[key]
) is not null. This filters out the keys with null values from the array.
Next, we use the reduce()
method to build a new object (filteredObj
) by iterating over the filtered keys. Inside the reduce function, we assign the key-value pairs to the accumulator object (acc
) if the value is not null.
Finally, we log the filteredObj
to the console, which will contain only the properties with non-null values.
By using this approach, you can effectively remove null values from an object by iterating and filtering its properties.
Iterating and Deleting
Another approach to remove null values from an object in JavaScript is by iterating over the object properties and deleting the properties with null values. Here’s an example demonstrating this method:
const obj = {
name: 'John',
age: null,
occupation: 'Developer',
address: null,
};
for (let key in obj) {
if (obj[key] === null) {
delete obj[key];
}
}
console.log(obj);
In this example, we define an object called obj
with properties that include null values.
We use a for...in
loop to iterate over the keys of the object. For each key, we check if the corresponding value (obj[key]
) is null. If it is null, we use the delete
keyword to remove that property from the object.
After the loop completes, the obj
object will no longer contain properties with null values. We can then log the modified obj
object to the console to see the result.
By utilizing this approach, you can remove null values from an object by iterating over its properties and deleting the properties with null values.
Creating A New Object
Another way to remove null values from an object in JavaScript is by creating a new object that excludes the properties with null values. Here’s an example:
const obj = {
name: 'John',
age: null,
occupation: 'Developer',
address: null,
};
const filteredObj = {};
for (let key in obj) {
if (obj[key] !== null) {
filteredObj[key] = obj[key];
}
}
console.log(filteredObj);
In this example, we start with an object called obj
that contains properties with null values. We initialize an empty object called filteredObj
to store the properties without null values.
We use a for...in
loop to iterate over the keys of the obj
object. For each key, we check if the corresponding value (obj[key]
) is not null. If it is not null, we assign the key-value pair to the filteredObj
object.
After the loop finishes, the filteredObj
object will only contain the properties from the original object that do not have null values. We can then log the filteredObj
object to the console to see the result.
By following this approach, you can create a new object that excludes properties with null values, effectively removing them from the original object.
Recursively Removing Nulls
When dealing with objects that have nested properties, you can recursively remove null values by traversing the object’s structure and eliminating any properties with null values at each level. Here’s an example of how you can achieve this:
function removeNulls(obj) {
for (let key in obj) {
if (obj[key] === null) {
delete obj[key];
} else if (typeof obj[key] === 'object') {
removeNulls(obj[key]); // Recursively call removeNulls for nested objects
if (Object.keys(obj[key]).length === 0) {
delete obj[key]; // Delete empty nested objects
}
}
}
}
const obj = {
name: 'John',
age: null,
occupation: 'Developer',
address: {
street: '123 Main St',
city: null,
country: null,
},
};
removeNulls(obj);
console.log(obj);
In this example, we define a function removeNulls
that takes an object obj
as a parameter. Within the function, we iterate over the object’s keys using a for...in
loop.
For each key, we first check if the value is null. If it is null, we delete the property from the object using the delete
keyword.
Next, we check if the value of the key is of type ‘object’, indicating that it is a nested object. In such cases, we recursively call the removeNulls
function on the nested object to remove null values within that object. We also check if the nested object becomes empty after removing null values. If it does, we delete the empty nested object from the parent object.
After executing the removeNulls
function on the initial object obj
, we log the modified obj
to the console. The result will be the object with all null values and empty nested objects removed.
Using this recursive approach, you can effectively remove null values from an object that contains nested properties, ensuring that all levels of the object structure are properly processed.
Using Libraries
To remove null values from an object in JavaScript, you can leverage libraries such as Lodash or Underscore.js. These libraries provide convenient methods to handle object manipulation tasks efficiently. Here’s an example using Lodash:
const _ = require('lodash');
const obj = {
name: 'John',
age: null,
occupation: 'Developer',
address: null,
};
const filteredObj = _.omitBy(obj, _.isNull);
console.log(filteredObj);
In this example, we import the Lodash library using require('lodash')
. Then, we have an object obj
with properties that include null values.
We use the _.omitBy()
method from Lodash to create a new object filteredObj
that excludes properties with null values. The first argument to _.omitBy()
is the object to filter, and the second argument is a predicate function _.isNull
that checks if a value is null. Any property with a null value will be excluded from the filteredObj
.
Finally, we log the filteredObj
to the console, which will contain only the properties without null values.
Underscore.js provides similar functionality with its _.omit()
method. You can refer to the Underscore.js documentation for more details on how to use it for removing null values from objects.
By utilizing these libraries, you can simplify the process of removing null values from objects in JavaScript and benefit from the utility functions they offer for object manipulation.
Conclusion
Removing null values from an object in JavaScript is a common task when working with data. Throughout this guide, we explored various approaches to achieve this goal effectively.
By iterating and filtering, we can selectively exclude properties with null values, creating a new object that contains only non-null values. The iterating and deleting approach allows us to remove null properties directly from the original object using the delete
keyword. Creating a new object by iterating and selectively assigning non-null properties provides a clean and filtered object representation.
For objects with nested properties, the recursive approach proves useful. By traversing the object structure and removing null values at each level, we ensure all nested levels are processed correctly.
Furthermore, we highlighted the option of using libraries like Lodash or Underscore.js, which provide dedicated methods for handling object manipulation tasks efficiently. These libraries offer convenience and flexibility, simplifying the process of removing null values from objects.
When applying these approaches, it is important to consider the specific requirements of your use case and the complexity of the object structure. Choose the method that best suits your needs in terms of performance, readability, and code maintainability.
By employing the appropriate approach, you can ensure your JavaScript code effectively removes null values from objects, improving the reliability and efficiency of your data processing.