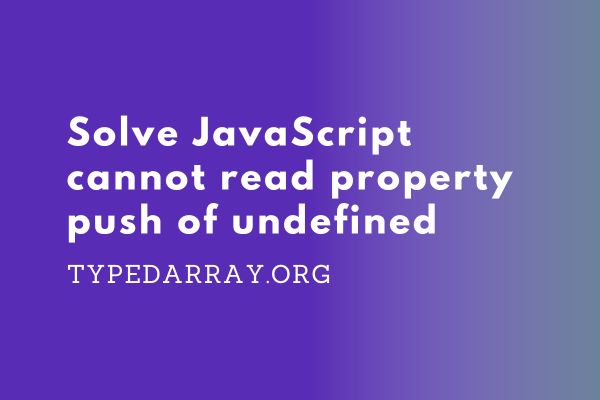
If you’ve tried to use the push method on an array in JavaScript and gotten an error message like “cannot read property ‘push’ of undefined,” don’t worry, you’re not alone.
This problem can be caused by a number of things from calling the push method on a variable that stores an undefined value to forgetting to initialize properties in a class before calling the push method on them.
In this blog post, we’ll explore some of the most common causes of this error message and offer solutions for how to fix it. So if you’re having trouble getting your arrays to work properly in JavaScript, keep reading!
Reasons Why You Get The ‘cannot read property push of undefined’ Error
The ‘cannot read property push of undefined’ error can occur because of these 10 main reasons –
1. You Call The push Method On A Variable That Is Undefined
2. You Forget To Initialize A Property In A Class
3. You Use The push Method On The Index Of An Array
4. Incorrect Data Type
5. Scope Issues
6. Asynchronous Code – Incorrect Timing
7. Misspelled Variable Or Object Name
8. Overwriting The Variable
9. The Object Is Null
10. Framework/library Issues
Let’s discuss each of these in detail below and look at some possible solutions.
1. You Call The push Method On A Variable That Is Undefined
This is the most common cause of the ‘cannot read property push of undefined’ error. It occurs when you try to call the push method on a variable that doesn’t exist or has an undefined value.
For example, consider the following code:
let myArray = [];
myArray.push("someValue"); // this will work fine
let anotherArray = undefined;
anotherArray.push("someValue"); // this will throw an error
In the above code, we first create a variable called myArray and initialize it with an empty array. We then call the push method on this variable and pass in ‘someValue’, so the push method will add the value ‘someValue’ to the end of our array.
Then we attempt to call the push method on anotherArray, which has an undefined value. Since the push method can only work on real JavaScript arrays and not variables that store undefined values, this will throw an error.
Solution: Make sure you’re calling the push method on a variable that has an actual array stored in it, instead of a variable with an undefined value.
You can use the Array.isArray method if you’re not sure whether or not a variable contains an array.
Here is an example:
let myArray = [];
if (Array.isArray(myArray)) {
myArray.push("someValue"); // this will work fine
} else {
console.log("myArray is not an array!");
}
You can also make sure that you initialize a variable to an empty array if you get that value from somewhere else, like a database.
const val = undefined;
const myArr = val || [];
myArr.push("someValue"); // this will work fine
Here, we have used the logical || operator to assign the value of val to myArr if val is undefined. If val has a defined value, then myArr will be assigned that value instead. But if val doesn’t have a defined value, we initialize it with an empty array.
2. You Forget To Initialize A Property In A Class
This second reason is a bit more specific and only applies if you’re trying to use the push method on an array that is a property of a class.
For example, consider the following code:
class MyClass {
constructor(values) {
this.values = values;
}
someMethod() {
this.myArray.push("someValue"); // this will throw an error
}
}
In the code above, we’ve created a class called myClass and we’ve initialized a property of the class called myArray. We then try to call the push method on this property, which will throw an error since it’s not an array.
var obj = new MyClass(["a","b"]);
obj.someMethod(); //TypeError: Cannot read property "push" of undefined
Solution: Make sure that you initialize a property in a class before you use it as an array. For example :
class MyClass {
constructor() {
this.myArray = []; // initialize the property here
}
someMethod() {
this.myArray.push("someValue"); // this will work fine
}
}
3. You Use The push Method On An Index Of An Array
The third and final reason for the ‘cannot read property push of undefined’ error is using the push method on an index of an array that doesn’t even exist.
For example, consider the following code:
let myArray = ["a","b","c"];
myArray[4].push("d"); // this will throw an error
In the code above, we’ve initialized myArray to be an array with three elements: ‘a’, ‘b’, and ‘c’. Then we try to call the push method on myArray’s fourth index, which doesn’t exist. This will cause JavaScript to throw an error since you can’t call the push method on a nonexistent index.
Solution: Don’t call the push function on an index of an array that doesn’t exist. For example:
let myArray = ["a","b","c"];
myArray.push("d"); // this will work fine
Note that you can still call the push function on myArray and pass in the index of ‘d’, since we’ve initialized myArray using real JavaScript arrays, which can have elements added to them.
4. Incorrect Data Type
One possible reason for the error “TypeError: Cannot read property ‘push’ of undefined” is when the variable or object you are trying to use the push
method on is not an array. The push
method is specifically used to add elements to an array, so if you attempt to use it on a variable or object of a different type, such as a string, number, or null, you will encounter this error.
For example, consider the following code snippet:
let myVariable = "Hello";
myVariable.push("world"); // Error: Cannot read property 'push' of undefined
In this case, myVariable
is a string, not an array. Since strings do not have a push
method, attempting to use push
on myVariable
will result in the “Cannot read property ‘push’ of undefined” error.
To resolve this issue, ensure that the variable or object you are using the push
method on is indeed an array. If the variable is not initially an array, you can initialize it as an empty array before using push
:
let myArray = [];
myArray.push("Hello");
console.log(myArray); // Output: ["Hello"]
In this updated example, myArray
is defined as an empty array. The push
method can now be used to add elements to myArray
without encountering the error.
5. Scope Issues
Scope issues can also lead to the “TypeError: Cannot read property ‘push’ of undefined” error in JavaScript. Here are a few scenarios where scope-related problems can occur:
- Incorrect variable scope: Ensure that the variable you are trying to access and use the
push
method on is within the correct scope. If the variable is defined within a function or block of code and you’re trying to access it from outside that scope, it will result in an undefined value. Verify that the variable is accessible from the current scope or consider moving the variable declaration to a higher scope if necessary.
Example:
function myFunction() {
let myArray = [];
// ...
}
console.log(myArray); // Error: Cannot read property 'push' of undefined
In this example, attempting to access myArray
outside the myFunction
scope will result in an error because the variable is not accessible in the outer scope.
- Timing issues in asynchronous code: If you’re working with asynchronous operations, such as callbacks or promises, the error can occur if the
push
operation is being performed at an inappropriate time. Ensure that the necessary data is available when thepush
operation is executed. Timing issues in asynchronous code can sometimes lead to undefined objects.
Example:
let myArray;
setTimeout(() => {
myArray.push("Hello"); // Error: Cannot read property 'push' of undefined
}, 1000);
In this example, the push
operation is scheduled to execute after a delay of 1000 milliseconds using setTimeout
. However, myArray
is not defined or initialized, causing the error.
To fix this, ensure that myArray
is defined and initialized before the asynchronous operation is executed:
let myArray = [];
setTimeout(() => {
myArray.push("Hello");
}, 1000);
Now, myArray
is properly defined and initialized as an array, and the push
operation can be executed without encountering an error.
Carefully examine your code and check for any scope-related issues, making sure that the variables and objects you’re accessing and using the push
method on are in the appropriate scope and accessible when needed.
6. Asynchronous Code
Asynchronous code can also be a potential cause for the “TypeError: Cannot read property ‘push’ of undefined” error. Here are a few scenarios where asynchronous code can lead to this error:
- Asynchronous function returning undefined: If you’re using an asynchronous function that returns a value, such as a Promise, and that function resolves to
undefined
, you may encounter this error when trying to access thepush
method on the returned value.
Example:
async function fetchData() {
// Perform asynchronous operations...
}
const data = fetchData(); // Assuming fetchData() returns undefined
data.push("Hello"); // Error: Cannot read property 'push' of undefined
In this example, if fetchData
returns undefined
, attempting to use the push
method on data
will result in the error. Ensure that the asynchronous function returns the expected data or handle the case where it may return undefined
.
- Asynchronous data retrieval: If you’re retrieving data asynchronously from an external source, such as an API, and attempting to push the data into an array, you need to make sure that the data is available before performing the
push
operation.
Example:
const myArray = [];
fetchDataFromAPI().then((data) => {
myArray.push(data); // Error: Cannot read property 'push' of undefined
});
console.log(myArray);
In this example, fetchDataFromAPI
retrieves data asynchronously, and the push
operation is performed inside the then
callback. However, if the console.log
statement is executed before the data is fetched and pushed into myArray
, the array will still be empty, leading to the error.
To address this, you need to ensure that the push
operation is performed when the data is available. You can consider using async/await
or chaining additional promises to synchronize the code execution.
- Incorrect scoping within asynchronous code: If you’re referencing variables within asynchronous callbacks or closures, ensure that the variables are accessible in the correct scope. If a variable is not properly scoped or if closures capture outdated values, it can result in undefined variables or objects.
Example:
let myArray;
setTimeout(() => {
myArray = [];
}, 1000);
myArray.push("Hello"); // Error: Cannot read property 'push' of undefined
In this example, the push
operation is executed immediately after the setTimeout
function, without waiting for the timeout to complete. Therefore, myArray
is still undefined when the push
operation is performed.
To handle this, you need to ensure that the push
operation is executed after the necessary asynchronous operation has completed:
let myArray;
setTimeout(() => {
myArray = [];
myArray.push("Hello");
}, 1000);
In this updated example, the push
operation is placed inside the setTimeout
callback, ensuring that myArray
is defined and initialized before the push
operation is executed.
When working with asynchronous code, it’s important to consider the timing of operations and ensure that the necessary data is available before performing any manipulations like the push
operation.
7. Misspelled Variable Or Object Name
Another potential reason for encountering the error “TypeError: Cannot read property ‘push’ of undefined” is when you misspell the variable or object name that you are trying to access. Misspelling the name will result in the variable being undefined, and attempting to use the push
method on an undefined variable or object will trigger the error.
Here’s an example that demonstrates this issue:
let myArray = [];
myArrayy.push("Hello"); // Error: Cannot read property 'push' of undefined
In this example, the variable myArray
is spelled incorrectly as myArrayy
when attempting to use the push
method. As a result, JavaScript will treat myArrayy
as an undefined variable, causing the error.
To fix this problem, double-check the spelling of the variable or object name and ensure that it matches the actual declaration or assignment. Correcting the spelling to match the intended variable or object will resolve the error:
let myArray = [];
myArray.push("Hello"); // Corrected spelling
In this updated example, the variable myArray
is spelled correctly, allowing the push
method to be called on the array without encountering the error.
It’s essential to review your code and verify that the variable or object name you are using is spelled correctly, ensuring consistency throughout your codebase.
8. Overwriting The Variable
Overwriting the variable or object with another value or assignment before calling the push
method can also cause the error “TypeError: Cannot read property ‘push’ of undefined.” Here’s an example to illustrate this issue:
let myArray = [];
myArray = 5;
myArray.push("Hello"); // Error: Cannot read property 'push' of undefined
In this example, myArray
is initially declared as an array and assigned an empty array []
. However, it is later overwritten with the value 5
. Since 5
is not an array and does not have a push
method, attempting to use push
on myArray
will result in the error.
To resolve this issue, ensure that you don’t inadvertently overwrite the variable or object with a different value or assignment before using the push
method. Make sure the variable maintains its intended type, such as an array in this case:
let myArray = [];
myArray.push("Hello"); // No error
In this corrected example, myArray
is properly defined as an array, allowing the push
method to be called without encountering an error.
Be cautious when assigning values to variables or objects, especially if they are expected to maintain a specific type or structure. Double-check your code to ensure that you’re not accidentally overwriting variables or objects with incompatible values before using methods like push
.
9. The Object Is Null
Another possible reason for encountering the error “TypeError: Cannot read property ‘push’ of undefined” is when the object you are trying to push into is explicitly set to null
. If the object is null, it does not have the push
method, and attempting to use push
on it will result in the error.
Here’s an example to illustrate this issue:
let myArray = null;
myArray.push("Hello"); // Error: Cannot read property 'push' of null
In this example, myArray
is explicitly assigned the value null
. Since null
is not an object and does not have a push
method, trying to use push
on myArray
will lead to the error.
To fix this problem, ensure that the object you are working with is properly initialized and not explicitly set to null
before using the push
method:
let myArray = [];
myArray.push("Hello"); // No error
In this corrected example, myArray
is initialized as an empty array, allowing the push
method to be used without encountering the error.
Always double-check that the object or variable you are working with is not null or explicitly set to null before attempting to access its properties or invoke methods on it.
Conclusion
In conclusion, encountering the error “TypeError: Cannot read property ‘push’ of undefined” in JavaScript can happen due to various reasons. Some common causes include incorrect data types, scope issues, misspelled variable or object names, overwriting the variable or object, issues with asynchronous code, and the object being null.
To avoid this error, it is essential to ensure that you are using the push
method on the correct data type, such as an array. Double-check the spelling of variable or object names to avoid undefined references. Be mindful of scope and ensure that variables are accessible from the current scope. Avoid overwriting variables or objects with incompatible values before using push
. When working with asynchronous code, ensure proper timing and availability of data. Lastly, verify that objects are not explicitly set to null before performing the push
operation.
By carefully reviewing your code, addressing these potential issues, and ensuring the correct usage of the push
method, you can prevent the occurrence of the “TypeError: Cannot read property ‘push’ of undefined” error and ensure smooth execution of your JavaScript programs.