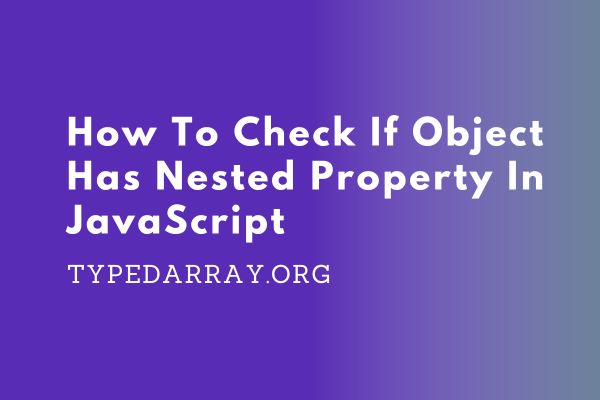
When working with JavaScript objects, it is often necessary to determine if a nested property exists within the object. Checking for the existence of nested properties is an essential task in many scenarios, such as validating data structures, accessing deeply nested values, or handling conditional logic based on property availability.
Fortunately, JavaScript provides multiple approaches to accomplish this task. In this guide, we will explore several methods to check if an object has a nested property. Whether you prefer using built-in operators, methods, newer language features, or custom utility functions, you will find a suitable technique to meet your specific requirements.
By understanding these different approaches, you can effectively handle nested property validation in your JavaScript code.
How To Check If Object Has Nested Property In JavaScript
There are many ways to check if an object has nested property in JavaScript. Some of these ways are –
- Using the in operator
- Using the hasOwnProperty() method
- Using optional chaining (introduced in ECMAScript 2020)
- Using the try…catch statement
- Using the typeof operator and nested checks
- Using a utility function that recursively checks for nested properties
These are just a few examples of how you can check for nested properties in JavaScript. The choice of approach depends on the specific requirements of your application and the JavaScript version you are using.
Let’s discuss each of these methods in detail below.
Using the in operator
The in
operator in JavaScript provides a straightforward way to check if an object has a nested property. By using the in
operator, you can determine if a specific property exists within an object, including nested properties. Here’s how it works:
if ('property1' in obj && 'property2' in obj.property1 && 'property3' in obj.property1.property2) {
// Property exists
}
In the above code snippet, we are checking for the existence of three nested properties: 'property1'
, 'property2'
, and 'property3'
. The in
operator is used to check if each property exists within its respective object. If all the properties exist, the condition evaluates to true
, indicating that the nested property is present.
This approach is simple and effective for checking nested properties. However, it is important to note that the in
operator also considers properties inherited from the object’s prototype chain. If you only want to check for properties directly present in the object itself, you may need to combine it with additional checks, such as obj.hasOwnProperty('property')
.
Using the in
operator provides a concise and readable way to validate the existence of nested properties in JavaScript objects.
Using the hasOwnProperty() method
In JavaScript, the hasOwnProperty()
method allows you to check if an object has a specific property, including nested properties. This method specifically checks if the property is a direct property of the object and not inherited from its prototype chain. Here’s how you can use hasOwnProperty()
to check for nested properties:
if (obj.hasOwnProperty('property1') && obj.property1.hasOwnProperty('property2') && obj.property1.property2.hasOwnProperty('property3')) {
// Property exists
}
In the above code snippet, we first check if the object obj
has a direct property 'property1'
using obj.hasOwnProperty('property1')
. If 'property1'
exists, we proceed to check if 'property2'
is a direct property of obj.property1
using obj.property1.hasOwnProperty('property2')
. Finally, we use obj.property1.property2.hasOwnProperty('property3')
to check if 'property3'
is a direct property of obj.property1.property2
. If all these conditions evaluate to true
, it indicates that the nested property exists.
By using hasOwnProperty()
, you can ensure that the properties being checked are not inherited from the object’s prototype chain, focusing only on the direct properties. This method provides a reliable way to validate the existence of nested properties in JavaScript objects.
Using optional chaining (introduced in ECMAScript 2020)
Introduced in ECMAScript 2020, optional chaining is a convenient feature in JavaScript that simplifies the process of checking for nested properties. It allows you to access nested properties without causing an error if any intermediate property is undefined or null. Here’s how you can use optional chaining to check for a nested property:
if (obj?.property1?.property2?.property3) {
// Property exists
}
In the above code snippet, the ?.
operator is used to chain the properties together. If any property in the chain is undefined or null, the expression short-circuits, and the overall result is undefined. This means that if any intermediate property does not exist, the condition will evaluate to false
, indicating that the nested property is not present.
The optional chaining syntax helps simplify the code and eliminates the need for manual checks at each level of nesting. It improves code readability and reduces the chance of encountering unexpected errors when accessing nested properties.
It’s important to note that optional chaining may not be supported in older versions of JavaScript engines or browsers. Therefore, be mindful of the environment in which your code will run and ensure that it supports ECMAScript 2020 or above if you choose to use optional chaining.
Using the try…catch statement
The try...catch
statement in JavaScript provides a way to handle exceptions and errors that may occur during the execution of a code block. It can also be used to check if an object has a nested property. Here’s how you can utilize the try...catch
statement for this purpose:
try {
if (obj.property1.property2.property3) {
// Property exists
}
} catch (e) {
// Property does not exist
}
In the above code snippet, we attempt to access the nested property obj.property1.property2.property3
within the try
block. If the property exists, the code within the try
block will execute normally. However, if any intermediate property is undefined or null, it will trigger an exception.
The catch
block is responsible for handling the exception. In this case, if an exception occurs, it means that the nested property does not exist. Within the catch
block, you can include code to handle the absence of the property, such as error logging or alternative logic.
Using the try...catch
statement can be useful when you expect the presence of a nested property but want to handle the case gracefully if it is not found. However, it’s important to note that relying on exceptions for control flow can have performance implications, so it is recommended to use this approach sparingly and when other methods of checking for nested properties are not suitable or available.
Using the typeof operator and nested checks
The typeof
operator in JavaScript allows you to determine the type of a value. By using the typeof
operator and nested checks, you can indirectly check if an object has a nested property. Here’s an example of how you can use the typeof
operator and nested checks to accomplish this:
if (typeof obj.property1 !== 'undefined' && typeof obj.property1.property2 !== 'undefined' && typeof obj.property1.property2.property3 !== 'undefined') {
// Property exists
}
In the above code snippet, we use the typeof
operator to check if each nested property exists. By comparing the result with 'undefined'
, we can determine if a property is defined or not. If any intermediate property is undefined
, the condition will evaluate to false
, indicating that the nested property is not present.
By performing nested checks using the typeof
operator, you can ensure that each level of the nesting is evaluated and that all properties exist before accessing the final property. This method provides a way to validate the existence of nested properties in JavaScript objects.
However, it’s important to note that the typeof
operator has some limitations. For example, it treats null
as an object and cannot distinguish between different object types. Therefore, this approach may not be suitable in all scenarios, especially when dealing with complex object structures or when the property you are checking can be of different types. In such cases, alternative approaches like using the in
operator or hasOwnProperty()
method may be more appropriate.
Using a utility function that recursively checks for nested properties
One effective approach to checking for nested properties in JavaScript objects is by using a custom utility function that recursively traverses the object’s properties. Here’s an example implementation of such a function:
function hasNestedProperty(obj, propertyPath) {
const properties = propertyPath.split('.');
let currentObj = obj;
for (let property of properties) {
if (!(property in currentObj)) {
return false;
}
currentObj = currentObj[property];
}
return true;
}
In the above code, the hasNestedProperty
function takes two parameters: obj
(the object to be checked) and propertyPath
(a string representing the nested property path, with each property separated by a dot).
The function first splits the propertyPath
string into an array of individual property names using the split()
method. Then, it iterates over each property name using a for...of
loop. Within the loop, it checks if the property exists in the current object using the in
operator. If the property does not exist, the function returns false
to indicate that the nested property is not present. If the property exists, the function updates the currentObj
variable to the value of the nested property, allowing the loop to proceed to the next level of nesting.
If the function completes the loop without encountering any missing properties, it means that the nested property is present, and the function returns true
.
You can use this utility function to check for nested properties as follows:
if (hasNestedProperty(obj, 'property1.property2.property3')) {
// Property exists
}
By utilizing a recursive utility function, you can perform deep checks for nested properties in JavaScript objects, making it a flexible and reusable approach for handling complex object structures.