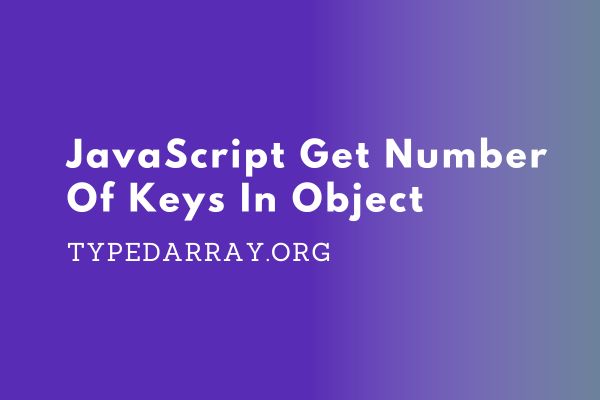
In JavaScript, objects are a fundamental data structure used to store key-value pairs. Sometimes, you may need to determine the number of keys (properties) present in an object. This can be useful for various purposes, such as iterating over the object, validating its structure, or analyzing its contents.
Thankfully, JavaScript provides several methods to obtain the count of keys in an object. By leveraging these techniques, you can effortlessly retrieve the number of keys and gain insights into the structure and size of your objects.
In this guide, we will explore different approaches to achieve this goal, empowering you to efficiently work with object properties in JavaScript.
JavaScript Get Number Of Keys In Object
There are many ways to get number of keys in object in JavaScript. Here are a few of them –
- Using Object.keys()
- Using Object.getOwnPropertyNames()
- Using Object.entries()
- Using a for loop
- Using Reflect.ownKeys()
- Get number of keys in inherited object
- Using Lodash
Let’s look at each of these methods in detail below –
Using Object.keys()
One of the ways to obtain the number of keys in an object in JavaScript is by using the Object.keys()
method.
The Object.keys()
method returns an array of a given object’s enumerable property names, which can then be used to determine the length or count of the keys.
Here’s an example:
const obj = { a: 1, b: 2, c: 3 };
const keysCount = Object.keys(obj).length;
console.log(keysCount); // Output: 3
In the code snippet above, we have an object obj
with three key-value pairs. By applying Object.keys(obj)
, we obtain an array containing the property names ["a", "b", "c"]
. We then use the .length
property on this array to determine the number of keys, which in this case is 3
. Finally, we log the result to the console.
By utilizing Object.keys()
, you can easily retrieve the number of keys in an object and leverage this information for various purposes in your JavaScript programs.
Using Object.getOwnPropertyNames()
Another method to get the number of keys in an object in JavaScript is by using the Object.getOwnPropertyNames()
method.
The Object.getOwnPropertyNames()
method returns an array of all properties (enumerable or non-enumerable) found directly on a given object. This array can then be used to determine the length or count of the keys.
Here’s an example:
const obj = { a: 1, b: 2, c: 3 };
const keysCount = Object.getOwnPropertyNames(obj).length;
console.log(keysCount); // Output: 3
In the code snippet above, we have an object obj
with three key-value pairs. By applying Object.getOwnPropertyNames(obj)
, we obtain an array containing the property names ["a", "b", "c"]
. We then use the .length
property on this array to determine the number of keys, which in this case is 3
. Finally, we log the result to the console.
Using Object.getOwnPropertyNames()
allows you to retrieve both enumerable and non-enumerable properties of an object, giving you a comprehensive count of keys present in the object.
Using Object.entries()
Another approach to determine the number of keys in an object in JavaScript is by utilizing the Object.entries()
method.
The Object.entries()
method returns an array of an object’s enumerable property key-value pairs, where each entry is represented as an array with two elements: the property key and its corresponding value. By obtaining this array, you can easily determine the length or count of the keys.
Here’s an example:
const obj = { a: 1, b: 2, c: 3 };
const keysCount = Object.entries(obj).length;
console.log(keysCount); // Output: 3
In the code snippet above, we have an object obj
with three key-value pairs. By applying Object.entries(obj)
, we obtain an array containing the property entries: [[ 'a', 1 ], [ 'b', 2 ], [ 'c', 3 ]]
. We then use the .length
property on this array to determine the number of keys, which in this case is 3
. Finally, we log the result to the console.
By utilizing Object.entries()
, you can easily access both the property keys and their corresponding values as an array, enabling you to determine the number of keys present in an object.
Using a for loop
You can also use a for loop to iterate over the keys of an object and count the number of keys present. Here’s an example:
const obj = { a: 1, b: 2, c: 3 };
let keysCount = 0;
for (const key in obj) {
if (obj.hasOwnProperty(key)) {
keysCount++;
}
}
console.log(keysCount); // Output: 3
In the code snippet above, we initialize a variable keysCount
to 0. Then, we use a for loop to iterate over each property in the object obj
. Inside the loop, we check if the object has the property (obj.hasOwnProperty(key)
) to exclude any inherited properties. If the property is found, we increment the keysCount
variable by 1. After the loop, we log the final count to the console.
Using a for loop allows you to manually iterate over the keys of an object and increment a counter variable, providing you with the total count of keys present.
Using Reflect.ownKeys()
Another method to obtain the number of keys in an object in JavaScript is by using the Reflect.ownKeys()
method.
The Reflect.ownKeys()
method returns an array of all property keys found directly on a given object, including both enumerable and non-enumerable properties. By obtaining this array, you can determine the length or count of the keys.
Here’s an example:
const obj = { a: 1, b: 2, c: 3 };
const keysCount = Reflect.ownKeys(obj).length;
console.log(keysCount); // Output: 3
In the code snippet above, we have an object obj
with three key-value pairs. By applying Reflect.ownKeys(obj)
, we obtain an array containing the property keys ["a", "b", "c"]
. We then use the .length
property on this array to determine the number of keys, which in this case is 3
. Finally, we log the result to the console.
Using Reflect.ownKeys()
allows you to retrieve all property keys of an object, including both enumerable and non-enumerable properties, giving you an accurate count of keys present in the object.
Using Lodash
If you have the Lodash library available in your JavaScript project, you can use the _.keys()
function to get the number of keys in an object. Lodash provides a convenient set of utility functions that can simplify common programming tasks.
Here’s an example of using _.keys()
from Lodash:
const _ = require('lodash');
const obj = { a: 1, b: 2, c: 3 };
const keysCount = _.keys(obj).length;
console.log(keysCount); // Output: 3
In the code snippet above, we import the Lodash library using the require()
function. Then, we have an object obj
with three key-value pairs. By applying _.keys(obj)
, we obtain an array containing the property keys ["a", "b", "c"]
using the Lodash utility. Finally, we use the .length
property on this array to determine the number of keys, which in this case is 3
. The result is logged to the console.
Lodash provides a comprehensive set of utility functions for working with objects, arrays, and more. The _.keys()
function is just one of many useful functions available in Lodash for manipulating and analyzing data structures.
Get number of keys in inherited object
To get the number of keys in an inherited object, you can combine the use of Object.keys()
and the for...in
loop. Here’s an example:
function Parent() {
this.a = 1;
this.b = 2;
}
function Child() {
this.c = 3;
Parent.call(this);
}
Child.prototype = Object.create(Parent.prototype);
const childObj = new Child();
let keysCount = 0;
for (const key in childObj) {
if (childObj.hasOwnProperty(key)) {
keysCount++;
}
}
console.log(keysCount); // Output: 3
In the code snippet above, we have a Parent
constructor function that defines two properties (a
and b
). The Child
constructor function extends Parent
and adds an additional property (c
). We create an instance of Child
called childObj
.
To count the keys, we iterate over the properties of childObj
using the for...in
loop. We use the hasOwnProperty()
method to check if each key is directly present in the object and not inherited from its prototype. If a key is found to be owned by the object, we increment the keysCount
variable. Finally, the count is logged to the console.
By combining Object.keys()
and the for...in
loop with hasOwnProperty()
, you can accurately count the number of keys in an object, including any inherited properties.
Conclusion
In conclusion, there are several ways to obtain the number of keys in an object in JavaScript. You can use methods like Object.keys()
, Object.getOwnPropertyNames()
, Object.entries()
, Reflect.ownKeys()
, or even a combination of for...in
loop and hasOwnProperty()
. Each approach provides a way to count the keys in an object, allowing you to gain insights into its structure and size.
The Object.keys()
method provides a straightforward solution, returning an array of enumerable keys that can be easily counted using the .length
property. Object.getOwnPropertyNames()
and Object.entries()
offer alternatives that consider both enumerable and non-enumerable properties.
If you need to handle inherited properties, the combination of a for...in
loop and hasOwnProperty()
ensures that only the object’s own keys are counted, excluding any inherited ones.
Additionally, if you have the Lodash library available, you can leverage its utility functions like _.keys()
to simplify the process of counting keys in an object.
By choosing the appropriate method based on your specific requirements, you can efficiently determine the number of keys in an object and utilize this information for further operations and analysis in your JavaScript code.