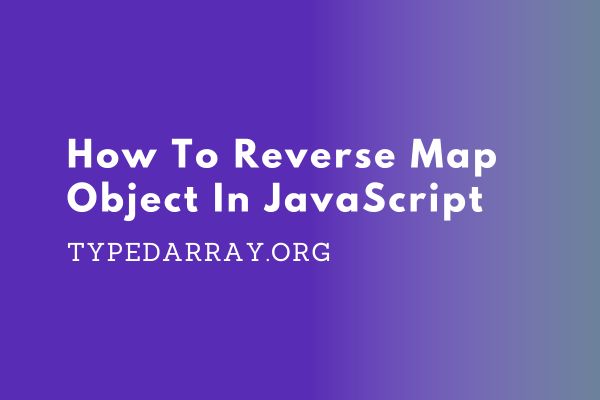
Reversing the mapping of an object in JavaScript refers to the process of swapping the keys and values of an object, creating a new object where the original values become keys and the original keys become values. This transformation can be useful in various scenarios, such as when you need to quickly access the original keys based on their corresponding values or perform operations that require a different lookup pattern.
In JavaScript, there are several approaches to achieve this reverse mapping, each offering its own implementation style and flexibility. By employing techniques such as iterating over object entries, utilizing reduce or map functions, or employing a for…in loop, you can effectively reverse map an object and obtain a new object where the keys and values have been interchanged.
These techniques provide developers with the ability to manipulate and work with objects in a versatile manner, adapting to their specific needs and requirements.
How To Reverse Map Object In JavaScript
There are several approaches to reverse map object in JavaScript. Some of these approaches are –
- Using a new object and iterating over the original object’s entries
- Utilizing
reduce
to create a new object - Leveraging the
map
function and spreading the result into a new object - Using a
for...in
loop to iterate over the object’s properties
Let’s discuss each of these methods in detail below.
Using a new object and iterating over the original object’s entries
One approach to reverse map an object in JavaScript is by using a new object and iterating over the original object’s entries. Here’s how it can be done:
function reverseMapObject(obj) {
const reversedObj = {};
Object.entries(obj).forEach(([key, value]) => {
reversedObj[value] = key;
});
return reversedObj;
}
const originalObj = { a: 1, b: 2, c: 3 };
const reversedObj = reverseMapObject(originalObj);
console.log(reversedObj); // { '1': 'a', '2': 'b', '3': 'c' }
In this approach, we create an empty object called reversedObj
. By using Object.entries(obj)
, we retrieve an array of key-value pairs from the original object. Then, we iterate over each entry using forEach
. For each entry, we assign the value as the key and the key as the value in the reversedObj
. Finally, we return the reversedObj
as the result.
By executing the example code above, you will obtain a new object reversedObj
where the original values from originalObj
become keys, and the original keys become values. In this case, the reversedObj
will be { '1': 'a', '2': 'b', '3': 'c' }
.
Utilizing reduce to create a new object
Another method to reverse map an object in JavaScript is by utilizing the reduce
function to create a new object. Here’s an example:
function reverseMapObject(obj) {
return Object.keys(obj).reduce((reversedObj, key) => {
reversedObj[obj[key]] = key;
return reversedObj;
}, {});
}
const originalObj = { a: 1, b: 2, c: 3 };
const reversedObj = reverseMapObject(originalObj);
console.log(reversedObj); // { '1': 'a', '2': 'b', '3': 'c' }
In this approach, we use Object.keys(obj)
to retrieve an array of keys from the original object. Then, we call reduce
on this array, providing an initial value of an empty object {}
.
Within the reduce
function, we iterate over each key. For each key, we assign the value as a key in the reversedObj
and the corresponding key as its value. The reversedObj
is accumulated and passed along in each iteration.
Finally, we return the reversedObj
, which contains the reversed mapping of the original object.
When you run the example code, you will obtain a new object reversedObj
where the original values from originalObj
become keys, and the original keys become values. The resulting reversedObj
will be { '1': 'a', '2': 'b', '3': 'c' }
.
Leveraging the map function and spreading the result into a new object
Another way to reverse map an object in JavaScript is by leveraging the map
function and spreading the result into a new object. Here’s an example:
function reverseMapObject(obj) {
return Object.fromEntries(
Object.entries(obj).map(([key, value]) => [value, key])
);
}
const originalObj = { a: 1, b: 2, c: 3 };
const reversedObj = reverseMapObject(originalObj);
console.log(reversedObj); // { '1': 'a', '2': 'b', '3': 'c' }
In this approach, we use Object.entries(obj)
to retrieve an array of key-value pairs from the original object. Then, we call map
on this array, which transforms each pair into a new array where the key becomes the value and the value becomes the key.
The resulting array of reversed key-value pairs is then passed to Object.fromEntries
, which creates a new object by spreading the array elements as key-value pairs.
Finally, we return the reversedObj
, which contains the reversed mapping of the original object.
When you execute the example code, you will obtain a new object reversedObj
where the original values from originalObj
become keys, and the original keys become values. The resulting reversedObj
will be { '1': 'a', '2': 'b', '3': 'c' }
.
Using a for…in loop to iterate over the object’s properties
One approach to reverse map an object in JavaScript is by using a for...in
loop to iterate over the object’s properties. Here’s an example:
function reverseMapObject(obj) {
const reversedObj = {};
for (let key in obj) {
reversedObj[obj[key]] = key;
}
return reversedObj;
}
const originalObj = { a: 1, b: 2, c: 3 };
const reversedObj = reverseMapObject(originalObj);
console.log(reversedObj); // { '1': 'a', '2': 'b', '3': 'c' }
In this approach, we create an empty object called reversedObj
. The for...in
loop iterates over each property of the original object obj
. For each property, we assign the value as a key in the reversedObj
and the corresponding key as its value.
Finally, we return the reversedObj
, which contains the reversed mapping of the original object.
By running the example code, you will obtain a new object reversedObj
where the original values from originalObj
become keys, and the original keys become values. The resulting reversedObj
will be { '1': 'a', '2': 'b', '3': 'c' }
.
Conclusion
Reversing the mapping of an object in JavaScript provides a way to interchange the keys and values of an object, enabling new lookup patterns and operations based on the transformed data structure. In this discussion, we explored several methods to achieve this reversal.
By iterating over the original object’s entries, we can create a new object where the values become keys and the keys become values. This can be done using techniques such as Object.entries
combined with iteration methods like forEach
or reduce
.
Alternatively, we can leverage the map
function and spread the resulting array of reversed key-value pairs into a new object using Object.fromEntries
. Another approach involves using a for...in
loop to iterate over the object’s properties and build a reversed object.
Each method provides a means to reverse map an object in JavaScript, allowing developers to adapt their data structures to different requirements and access patterns. By employing these techniques, you can effectively transform an object, facilitating tasks such as value-based lookups or performing operations based on the reversed mapping.